Building a webcall for customer support: How to make a call fallback web app for Freshdesk
Introduction: Building a webcall for customer support
Customer support over chat is efficient, but there are times when a chat support agent may need to continue the conversation over a call. The easiest way to do so is to invite the customer to a webcall.
What is a webcall?
A webcall is a voice or video call conducted through a web-based platform like Sendbird. It allows people to communicate in real-time via their browser without the need to install any apps. It allows people in different geographic locations to connect with one click via a website and communicate as if they were in the same room. In this tutorial, the use case consists of a support agent sending a web link to a customer to join him on a video call.
This tutorial outlines how to build a service that creates a:
A virtual room to host a group call using the Sendbird Calls API
Service user and their temporary authentication token
URL for opening a hosted Sendbird video call web app
This tutorial will also show how to:
Generate a URL that opens the Sendbird-enabled voice and video call web app
Create a simple web app to host a voice and video call
Why should you allow your customer to get on a call from their browser?
The main reason why a support chat agent would connect with a customer via a webcall is simplicity. Webcalls work across devices and operating systems and only require the delivery of a simple URL via chat. Their simplicity and versatility ensure that the support agent can always connect with their customer. As a result, they are a great fallback service.
What is a fallback service?
A fallback service is a secondary service that can be used as a backup when the primary service is unavailable or fails to respond within a specified time period. The fallback service is intended to provide a temporary solution until the primary service becomes available again. The goal is to ensure that the user's experience is not negatively impacted by service downtime or failure.
To operate correctly, the URL string needs to include the following parameters:
A Sendbird Group Call room ID
A User ID and a short-lived authentication token of a service user
- Password encrypted params (optional)
A password would need to be passed to the end user via a messaging service.
After receiving the URL, the end user or merchant will:
Click a link and open a web browser that hosts a voice and video call web app
Copy a password into the hosting site
Connect to the Sendbird-enabled call app using the credentials provided to them by a service user
first, let’s talk briefly about service users in the context of this tutorial.
What is a service user?
A service user is a term commonly used in software or web applications to refer to a user account used by a system or application to perform specific tasks or functions. Service users are often created by system administrators or developers to provide a level of separation between the application and the end user. A user-generated in Sendbird associated with the end user/merchant, but not the actual end user/merchant, is a service user.
Why is a service user needed?
The end user or merchant needs a user_id and credentials to enter a group call in this fallback service. Providing an agent with the ability to generate credentials for a real end user/merchant account is not recommended because it could lead to a breach of security and improper use of Group Calls and of Sendbird.
How is a service user created?
A service user is created using Sendbird’s Platform API:
Create a new associated end user/merchant. For example, with the user_id - service_user_30400606506004.
Get a new short-lived session token.
Pass the user_id and token to the end user/merchant as a URL link.
Use the same end user/merchant service user again in the future as needed.
Now that we understand the basics let’s talk about how to use Sendbird’s Platform API for a Proof Of Concept (POC) and in production.
How can Sendbird’s Platform API be used for web calling?
POC - Agent’s side
- Freshdesk
Use Freshdesk’s backend request method. The Freshdesk code is described later in this tutorial.
- Android and iOS
Pass a Sendbird API token to the app and use an Android network request or iOS Alamofire to Sendbird’s Platform API.
WARNING! Calling the Sendbird Platform API directly from a user’s client is not secure. This is strictly NOT for use in production.
Production
- Freshdesk
Use Freshdesk’s backend request method, which is the same as in the POC
- Android and iOS
Put a proxy server between the client and Sendbird. Securly call the proxy server from Android and iOS. Forward the call to Sendbird’s Platform API, and then return the result to the Android and iOS clients.
With the foundation built, let’s now talk about the implementation of a webcall fallback!
Webcall fallback implementation flow
The diagrams below depict the flow for Freshdesk, a POC for a field agent client, and a production flow for a field agent client. Note the presence of the proxy server in the production flow.
Flow diagram: Freshdesk
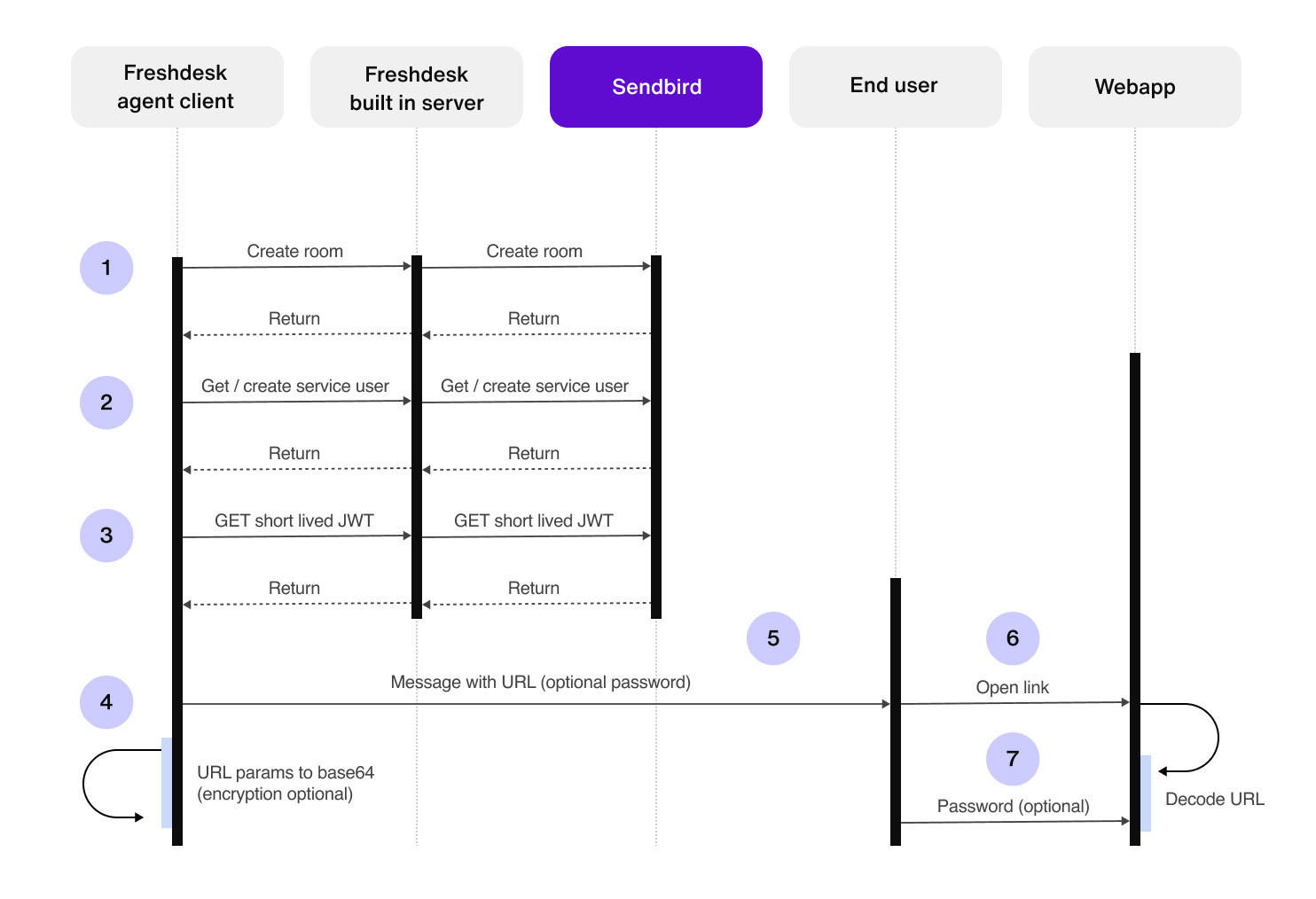
Flow diagram: POC field agent client
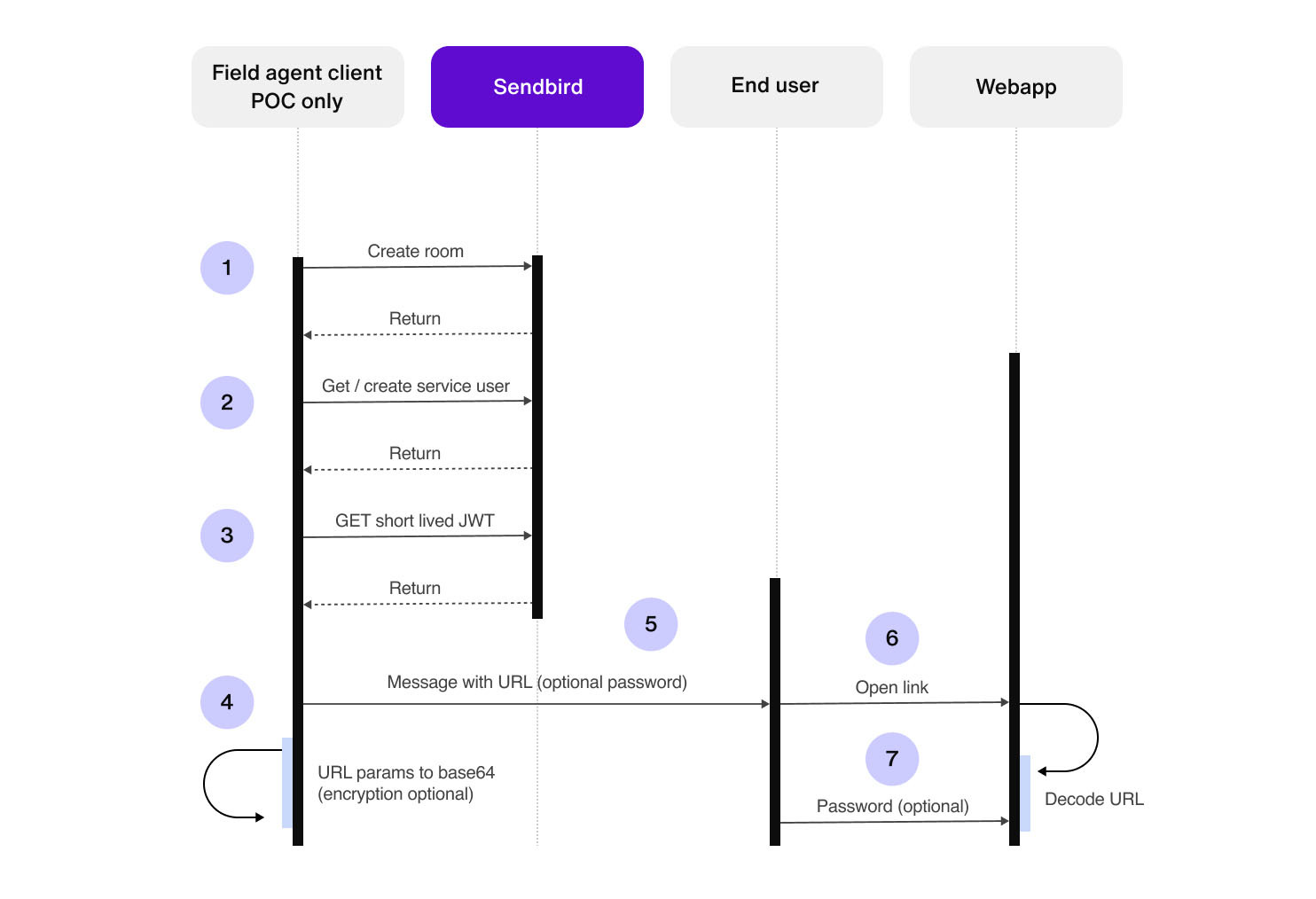
Flow diagram: Production field agent client
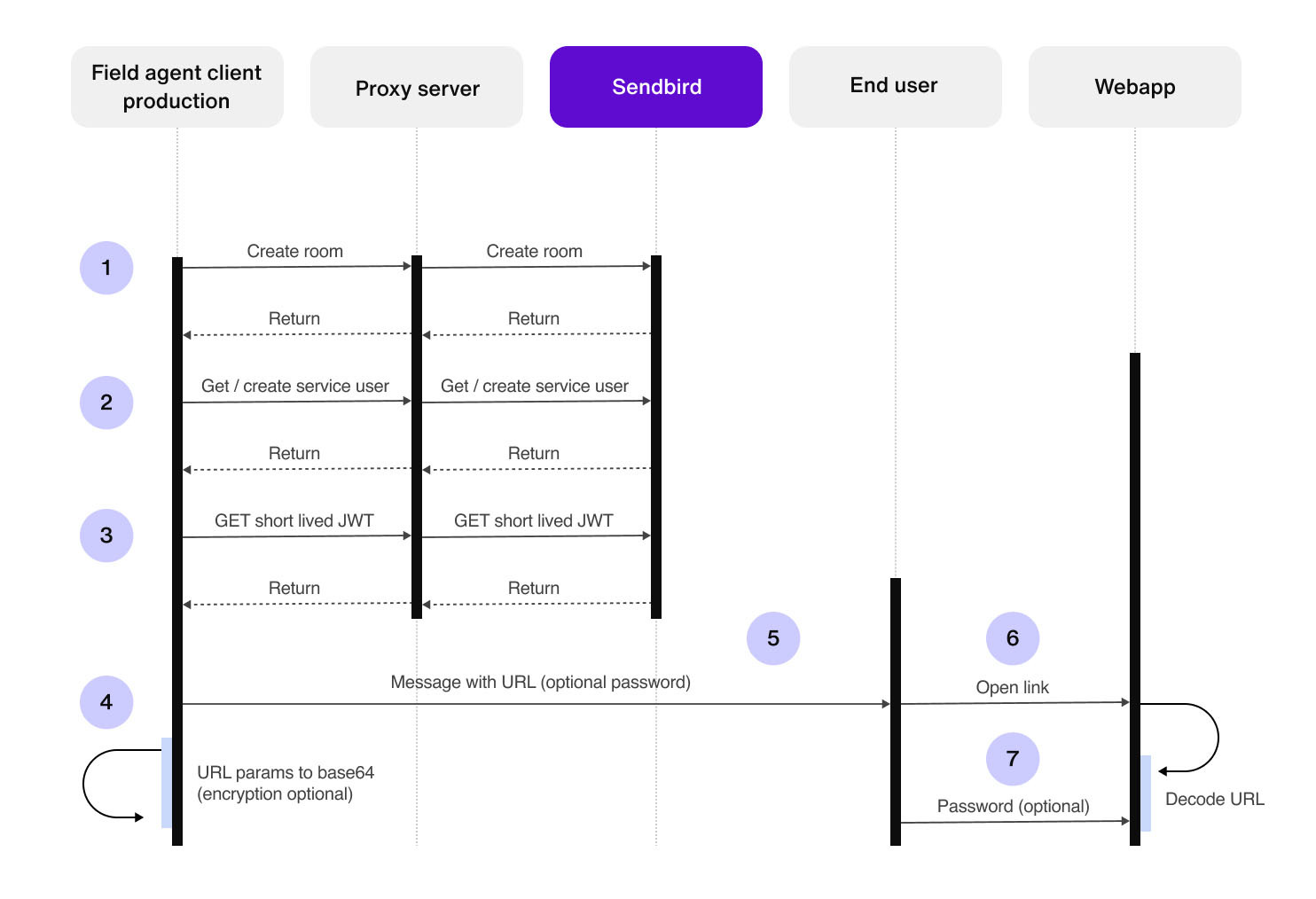
Here’s what happens in the production flow for web calling:
The agent creates a new room via their frontend or backend.
The agent’s backend checks if there is a service user and creates a service user if none exists (potentially try to generate a session token first, and if the user doesn’t exist, then create one).
Agent requests a 1-hour session token.
- Agent generates a URL with the params encoded to base64.
- Encrypted params include:
The service user’s ID
The service user’s session token and expiry
The Room ID
- Encrypted params include:
Optionally, the base64 encoded params could be the password(salt) encrypted using some cross-platform encryption.
The agent sends the URL as a message to the end user (sends password(salt) separately if needed).
The end user clicks the URL link (enters password if used).
- The URL’s encrypted params are decrypted.
The Room ID is known, the user ID is known, the session token is known.
The end user can enter the Sendbird room.
Webcall implementation: Using Freshdesk’s backend as a proxy server
To run the sample server code, you can clone the repository, open the terminal, and run the Freshdesk FDK command. Please see this GitHub repo for the code for the Freshdesk backend.
The manifest in this system is a set of methods that allow Freshdesk's backend to communicate with the Sendbird platform. One of these methods, getAgentsSendbirdCreds, retrieves an agent's Sendbird credentials with a single call. Another method, buildBackupGroupCallUrl, handles the logic and API calls needed to create a Sendbird Group Calls room, service user, and fetch the service user's credentials.
The file app.js is connected to the widget user interface in Freshdesk and makes calls to server.js to get an agent's Sendbird credentials. It then passes ticket details and the agent's credentials to modal.js.
modal.js handles the modal UI and gathers data from server.js to create a shareable URL. The modal displays buttons to request the URL and displays the Sendbird Group Call room data stream.
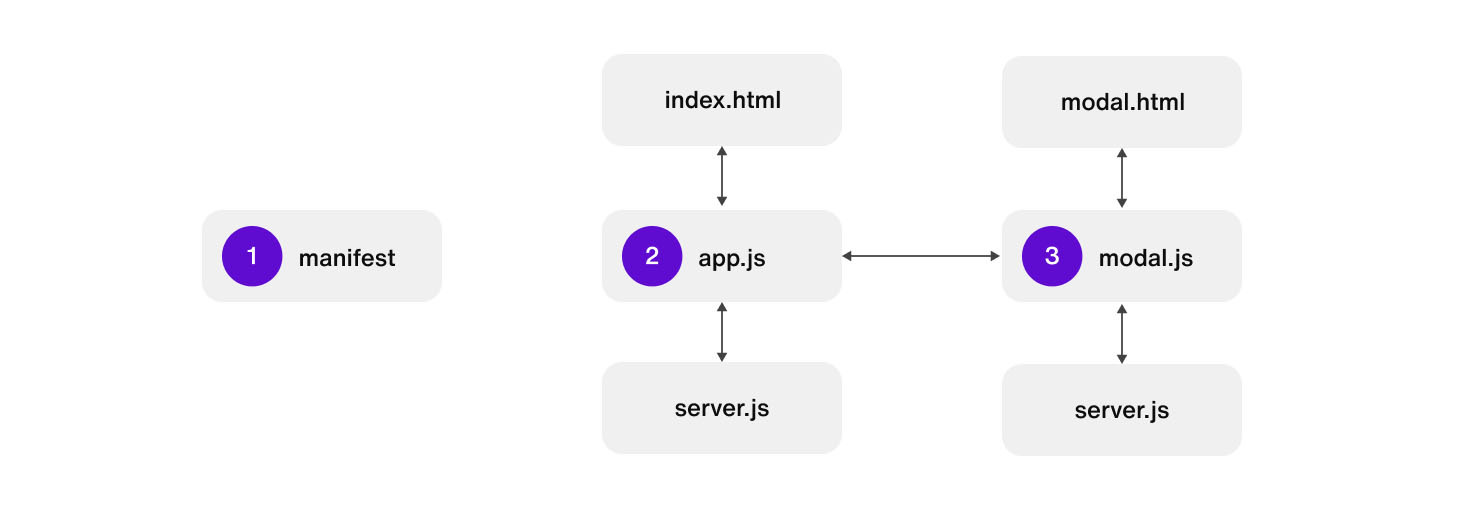
Freshdesk flow
Step 1
In manifest.js, register two methods for calling from Freshdesk to Sendbird.
Step 2
In app.js, prepare to gather the ticket details and call to Sendbird for the agent’s Sendbird credentials.
server.js exports.getAgentsSendbirdCreds
Note: renderData is a native method of FreshDesk and returns from the function call.
Step 3
When the openModal()
button is clicked, collect the ticket details and agent credentials. Pass the data from app.js to modal.js, then open the modal.
Step 4
The modal opens and provides a button for the agent to click to create a shareable Sendbird Group Calls URL. The URL opens the hosted web app outlined later in this document. But, first ingest any incoming data from app.js.
Step 6
The user calls to create a new URL to share with the merchant. The URL is encoded to base64 and displayed to the agent.
Now, make a call to fetch create a new room and provide a service user with credentials.
Business logic:
- Try to fetch credentials for a service user.
If you succeed, go to step 2.
If failed, create the user and start step 1 again.
Create Sendbird Group Call room.
server.js - Helper methods
Step 7
The agent should share the URL with the merchant and then enter the Sendbird Group Call room.
Add room listeners to check when the merchant is connected, and then display the merchant’s video in the modal UI.
Conclusion: Building a web call fallback fast for Your Freshdesk support agents
Congratulations! You have successfully implemented a web video call! In this tutorial, we created a group call room, generated a service user with their temporary authentication token, and created a URL for opening a hosted Sendbird web app for making a Sendbird video call.
Don’t forget to check out the code on GitHub for the Freshdesk backend. If you need help, the Sendbird Community is a great place to start. You can also visit the docs to learn more about implementing Sendbird Calls. If you still have questions, don't hesitate to contact us! Our experts are always happy to help.
Happy web calls fallback building! 🖥