Build in-app chat, voice & video Build in-app notifications Build in-app AI chatbots Build in-app communications
Save a ton of engineering bandwidth using a proven, reliable, and scalable API platform.
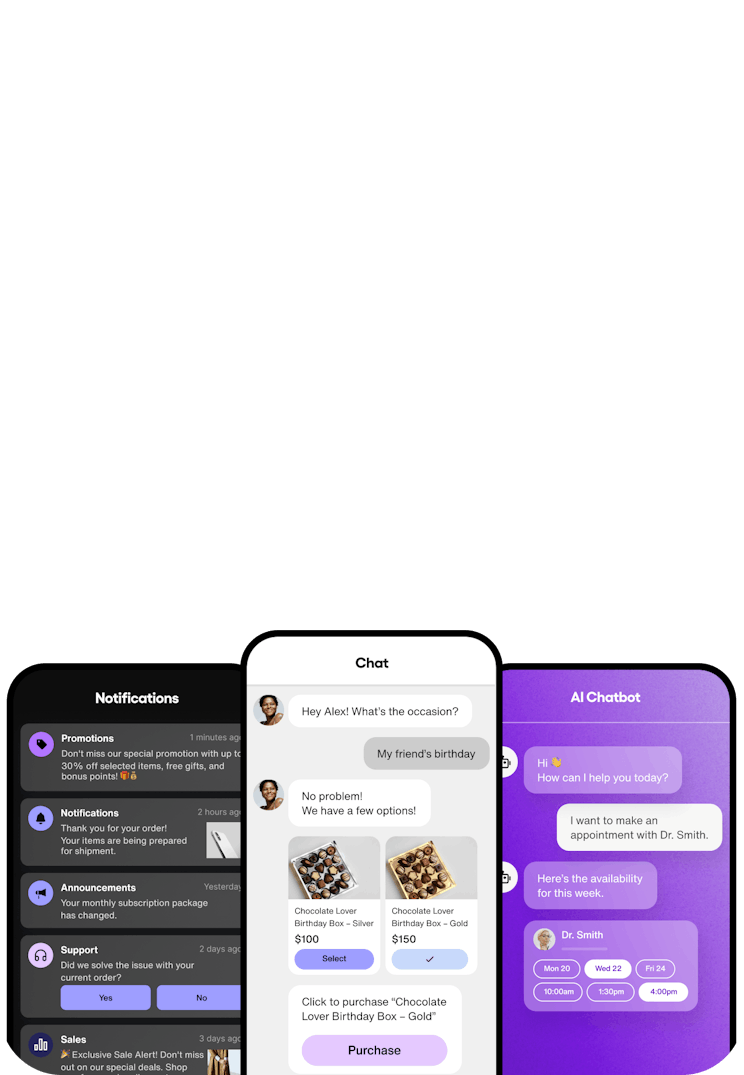
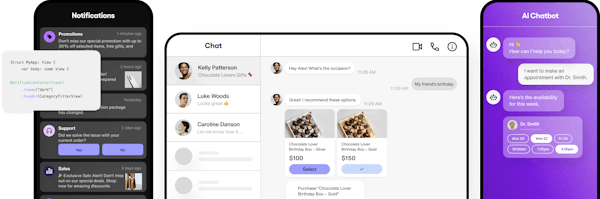
TRUSTED BY 4,000+ APPS GLOBALLY
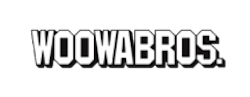
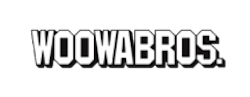
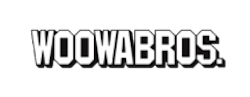
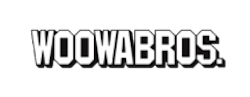
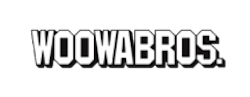
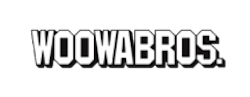
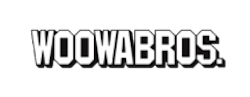
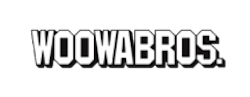
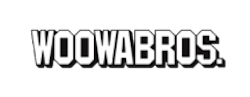
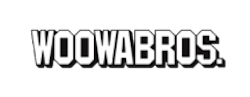
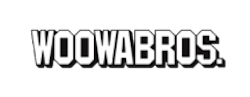
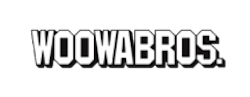
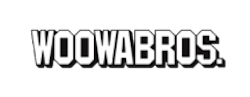
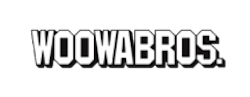
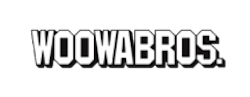
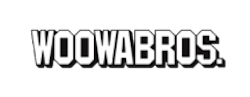
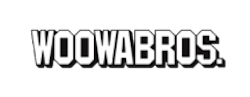
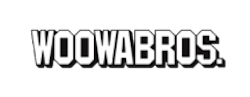
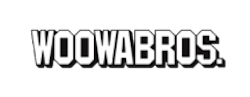
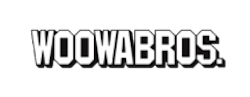
Award-winning communications platform
Named G2 leader and high performer
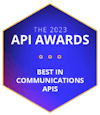
Awarded best in Communications APIs
11 cloud regions powered by AWS
300 million+ monthly active users
145,000+ global developers
7 billion+ messages per month
Choose the best plan for your business
Futureproof mobile engagement with a WhatsApp-like experience
Futureproof mobile engagement with a WhatsApp-like experience
Engage, convert, and support your customers
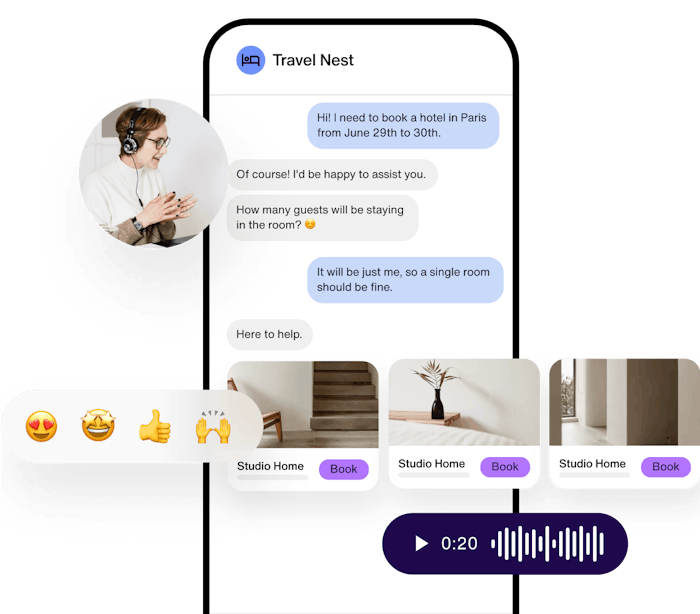
Customer retention
Leverage Sendbird APIs and SDKs to build customized in-app chat, voice, video and live streaming experiences that engage your users in real-time to keep them coming back to your app for more.
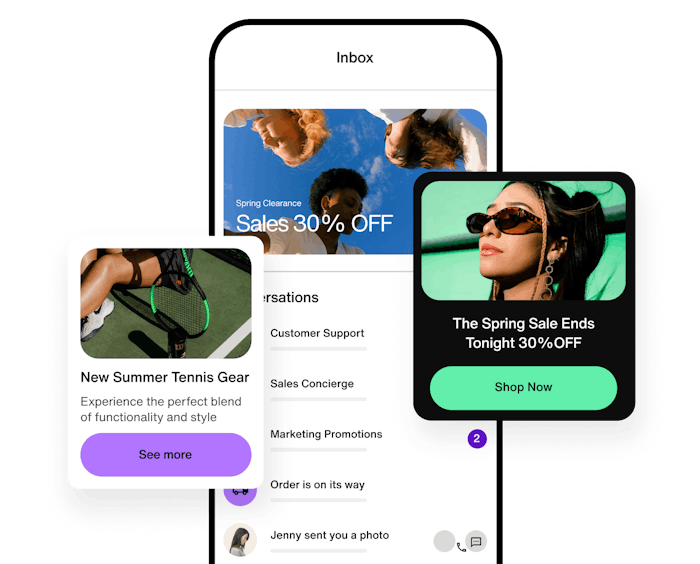
Customer conversion
Reach customers with messages that persist in your app. Scalable, secure, and fast-to-launch, Sendbird Notifications convert at a 2X higher rate than SMS alone, for half the cost.
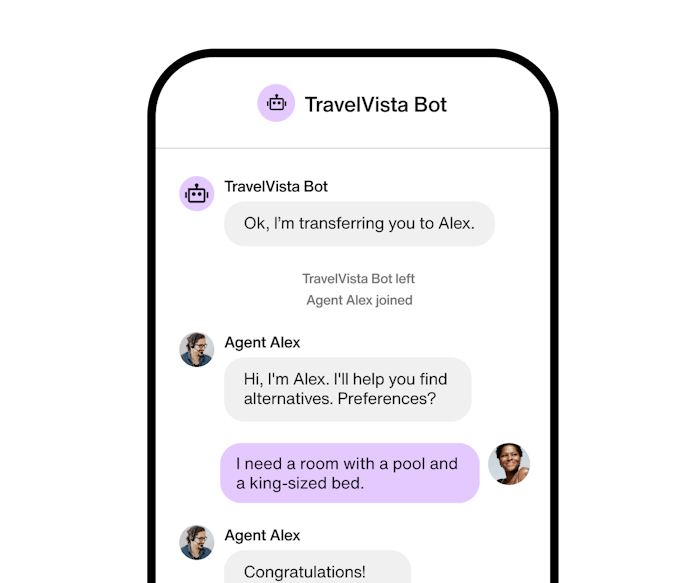
Customer satisfaction
Provide top-notch customer service with the perfect combination of human touch and artificial intelligence. Expand your CRM or CS solution to provide real-time chat support directly to users within your app using Sendbird integrations.
Sendbird’s solution for every industry
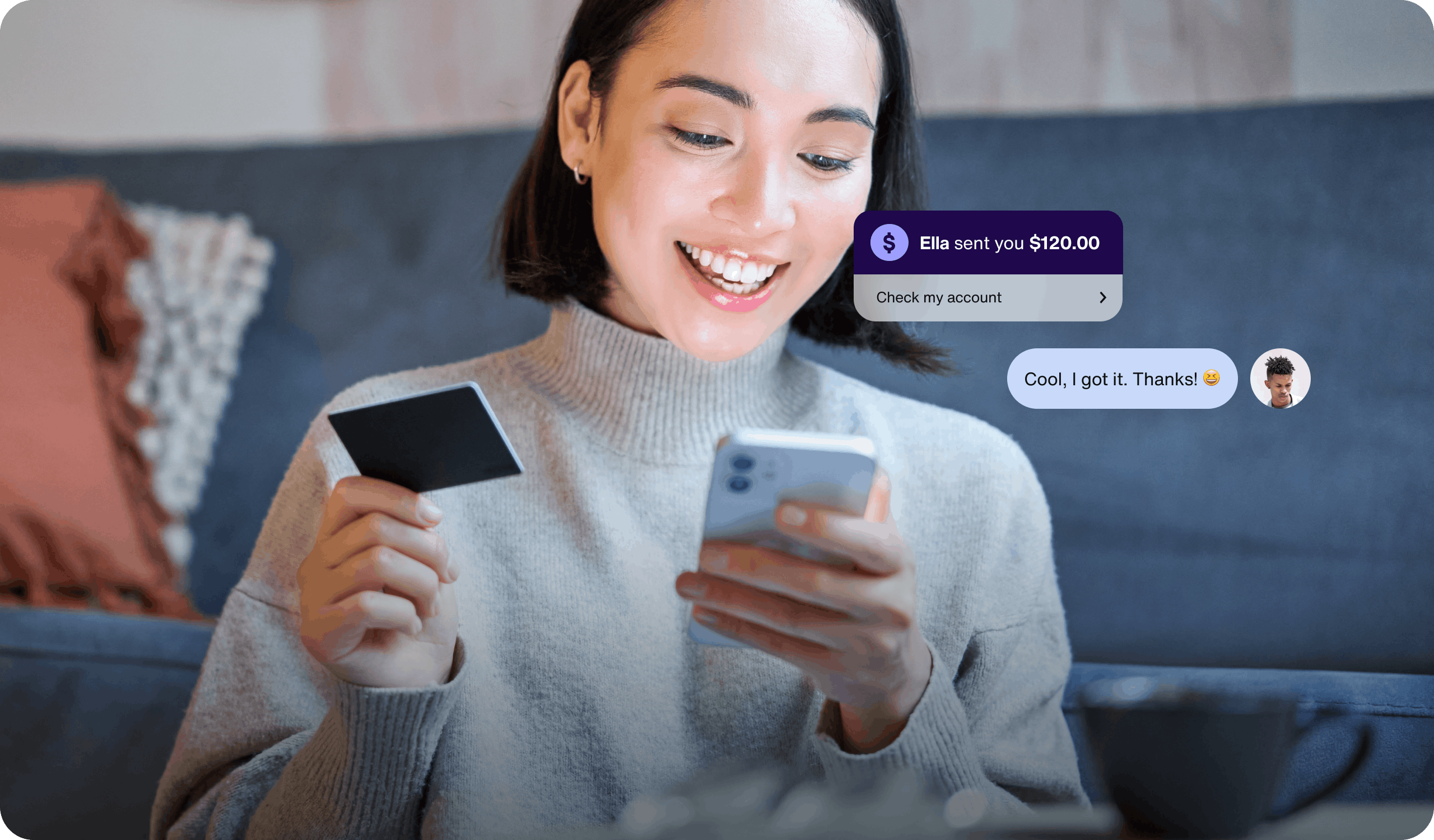
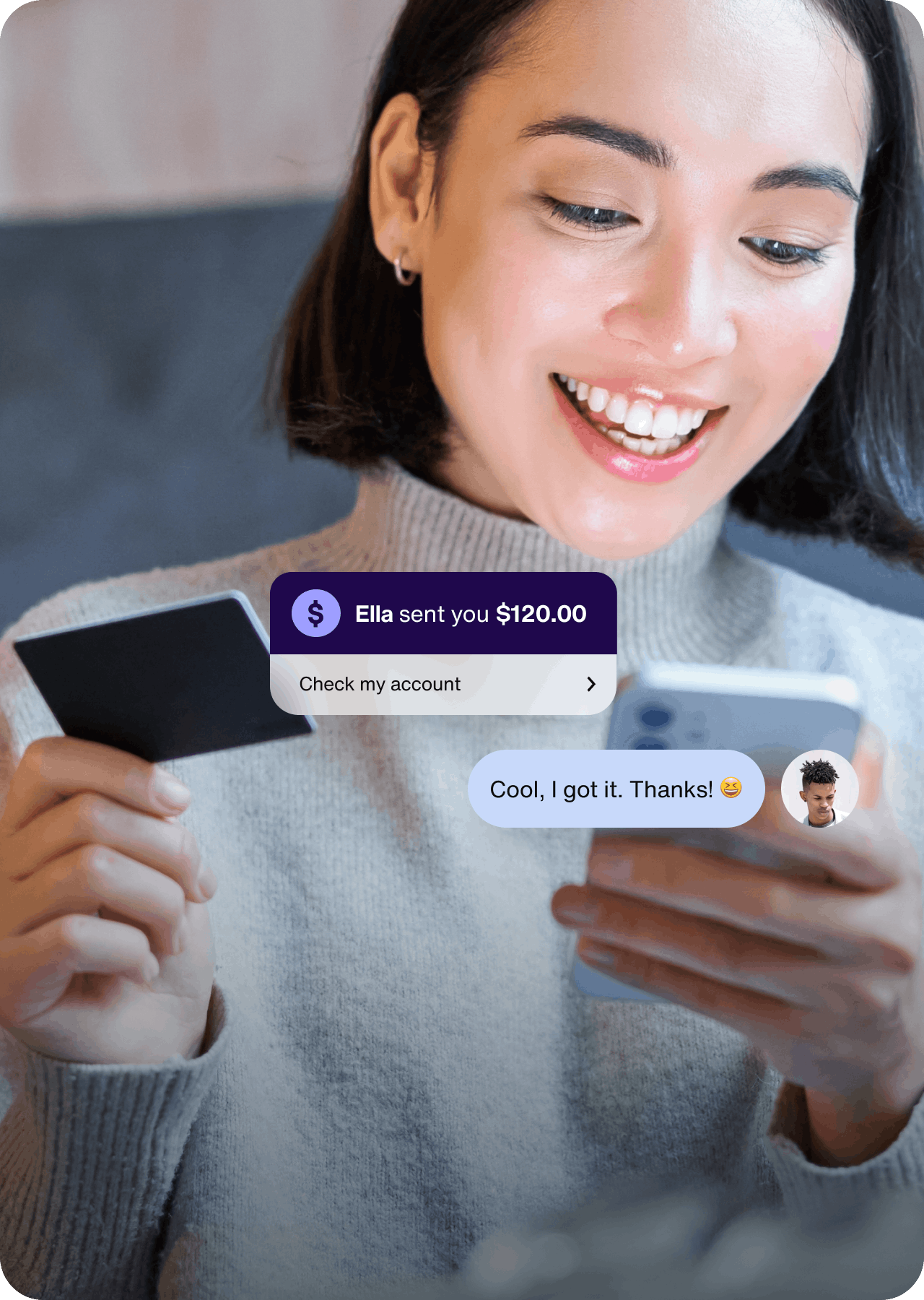
Turn a financial transaction into a lasting relationship.
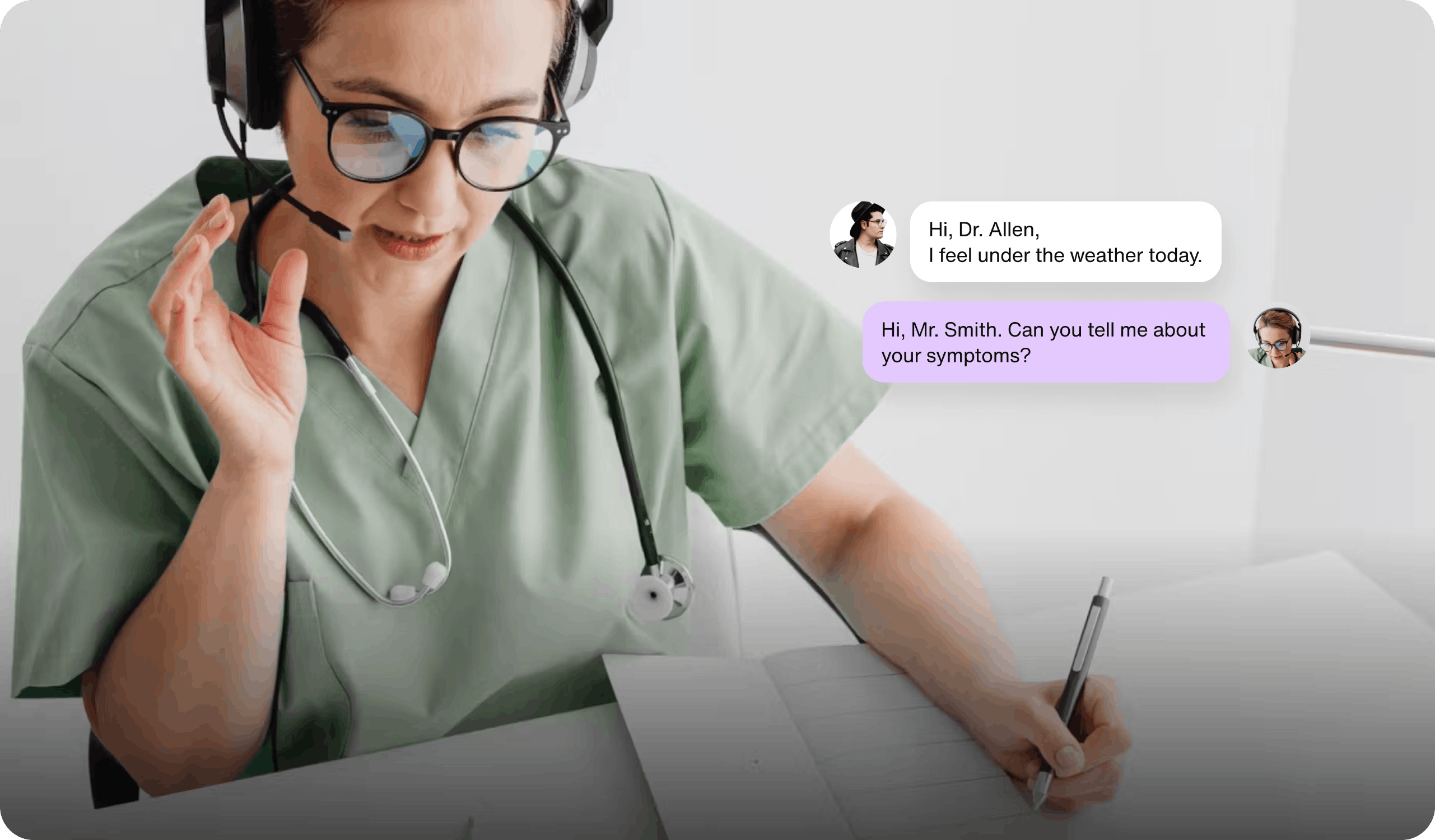
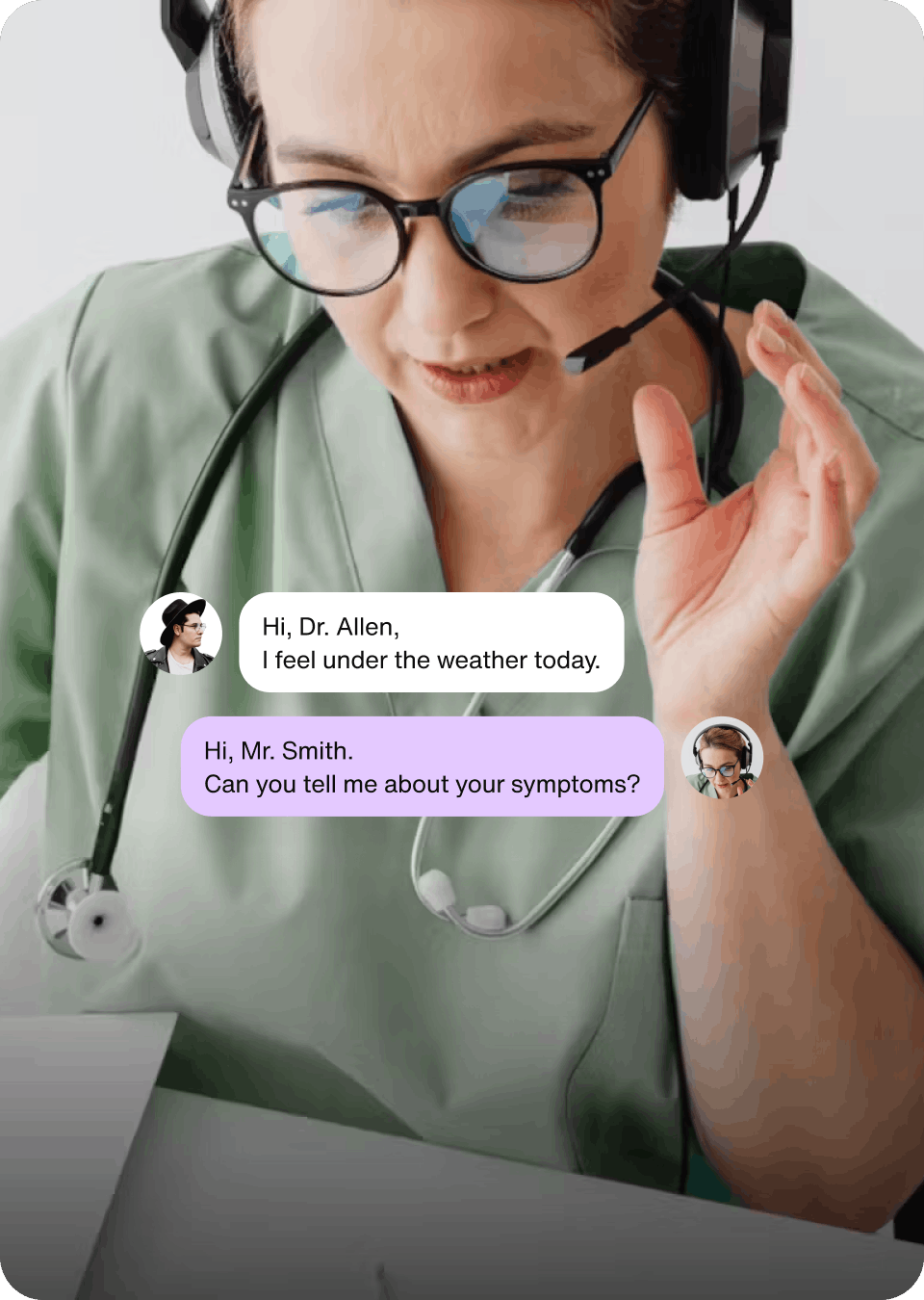
Build immersive chat, voice, and video experiences for the modern connected patient. Be there for your patients at every step of their journey.
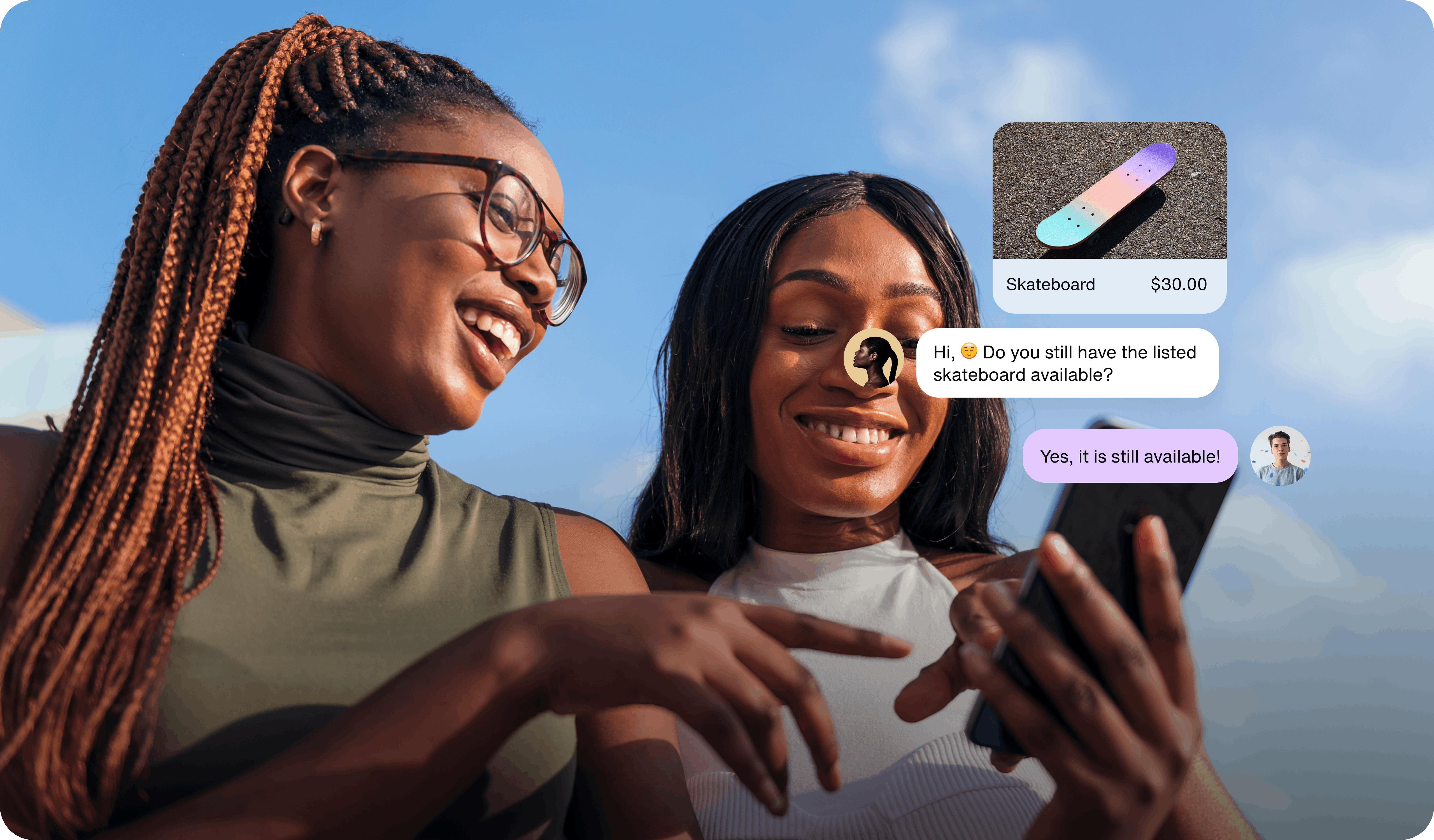
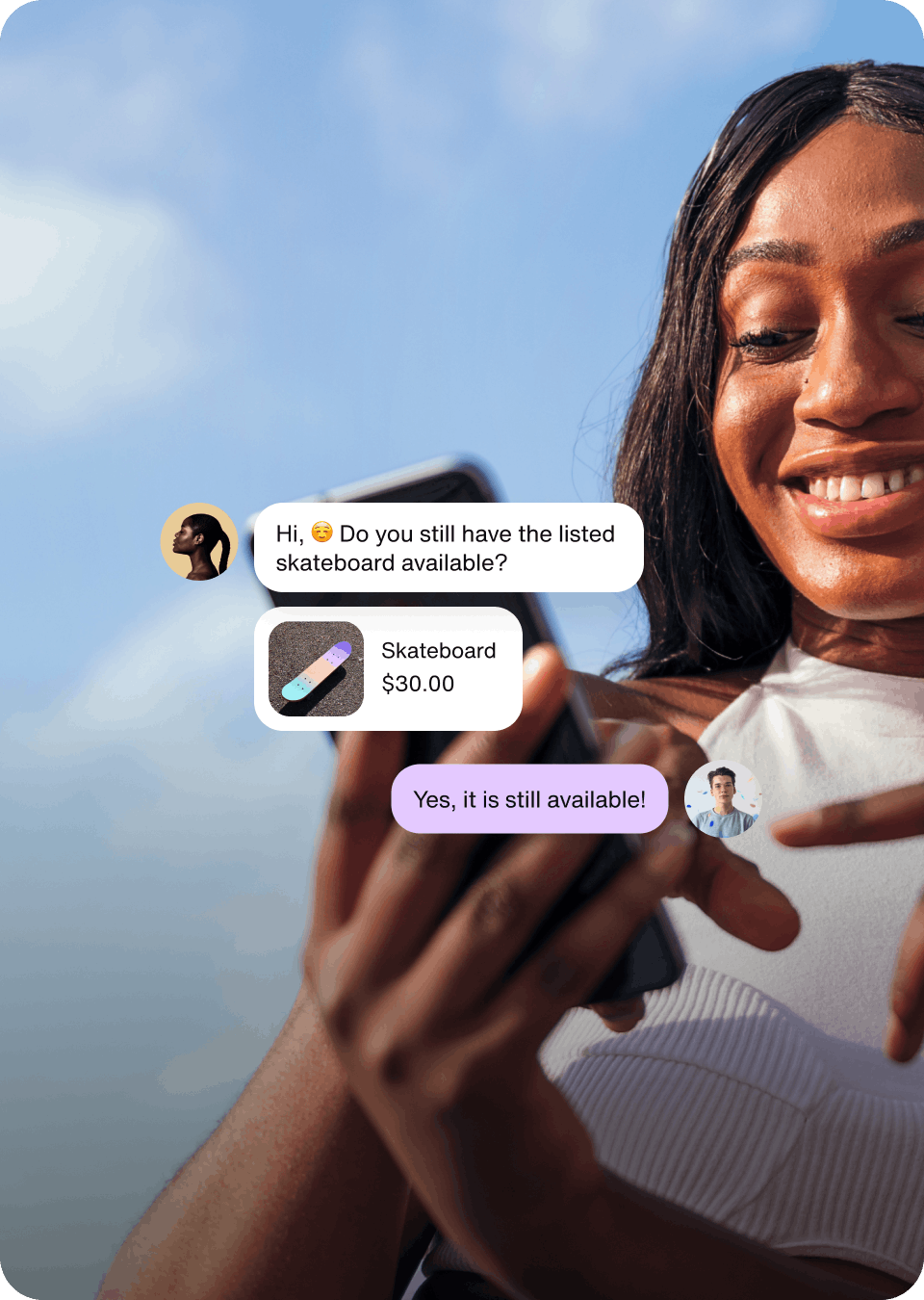
Connect buyers and sellers, and drive more transactions. Integrate chat with your shopping cart, payment system, reviews platform, and more.
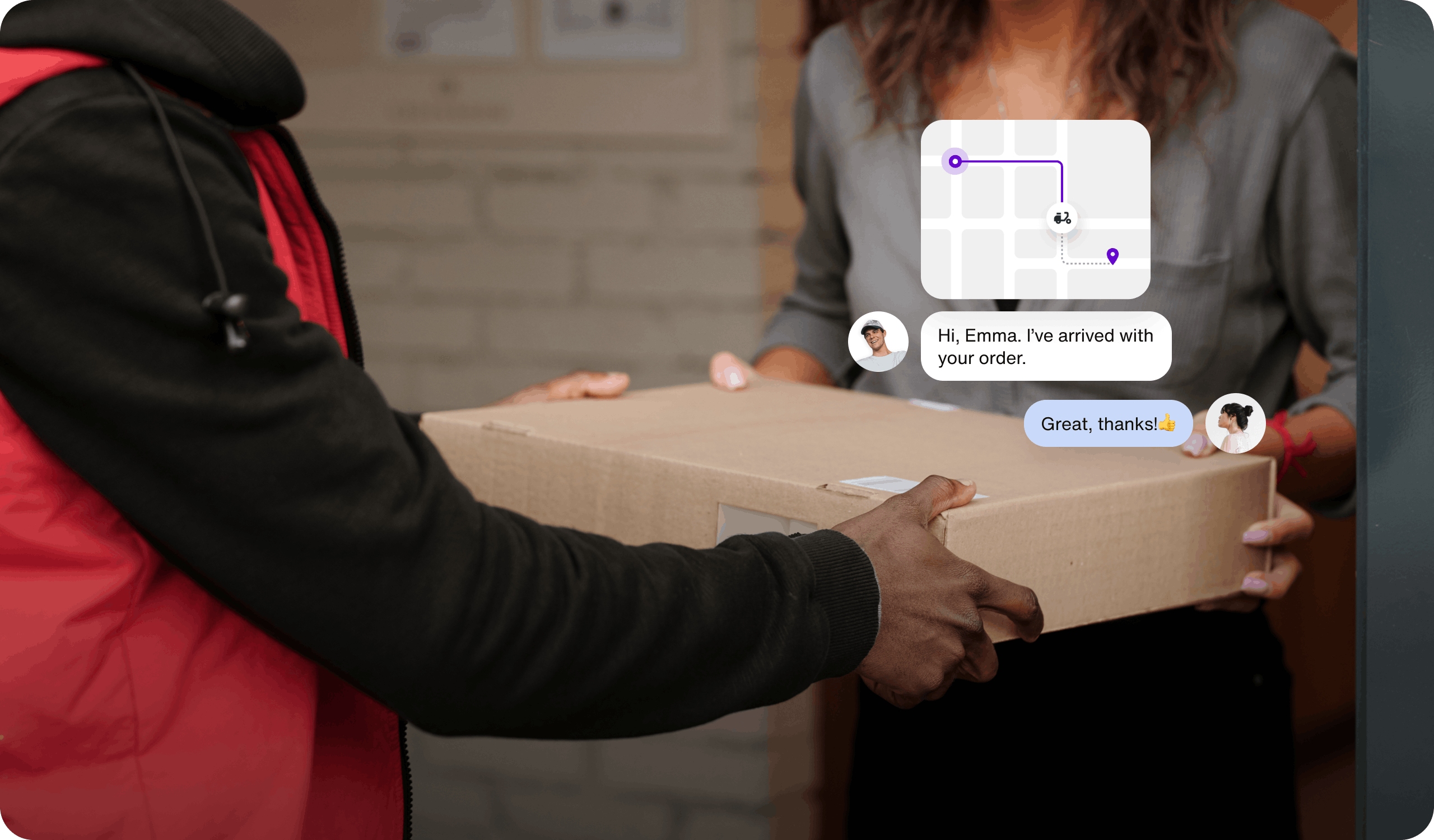
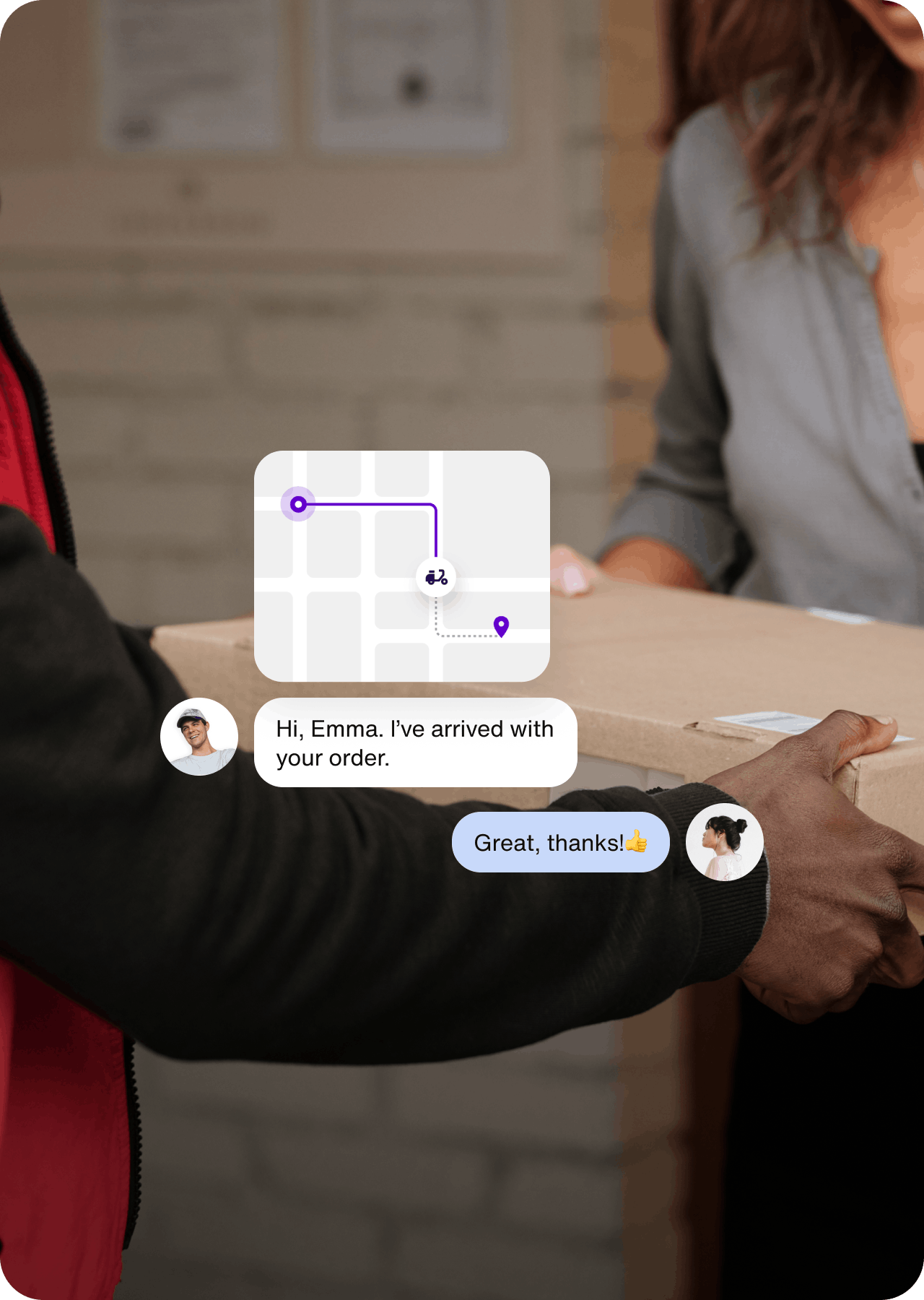
Be it ride sharing or delivery, prevent booking cancellations and missteps through always-on communication between your customers.
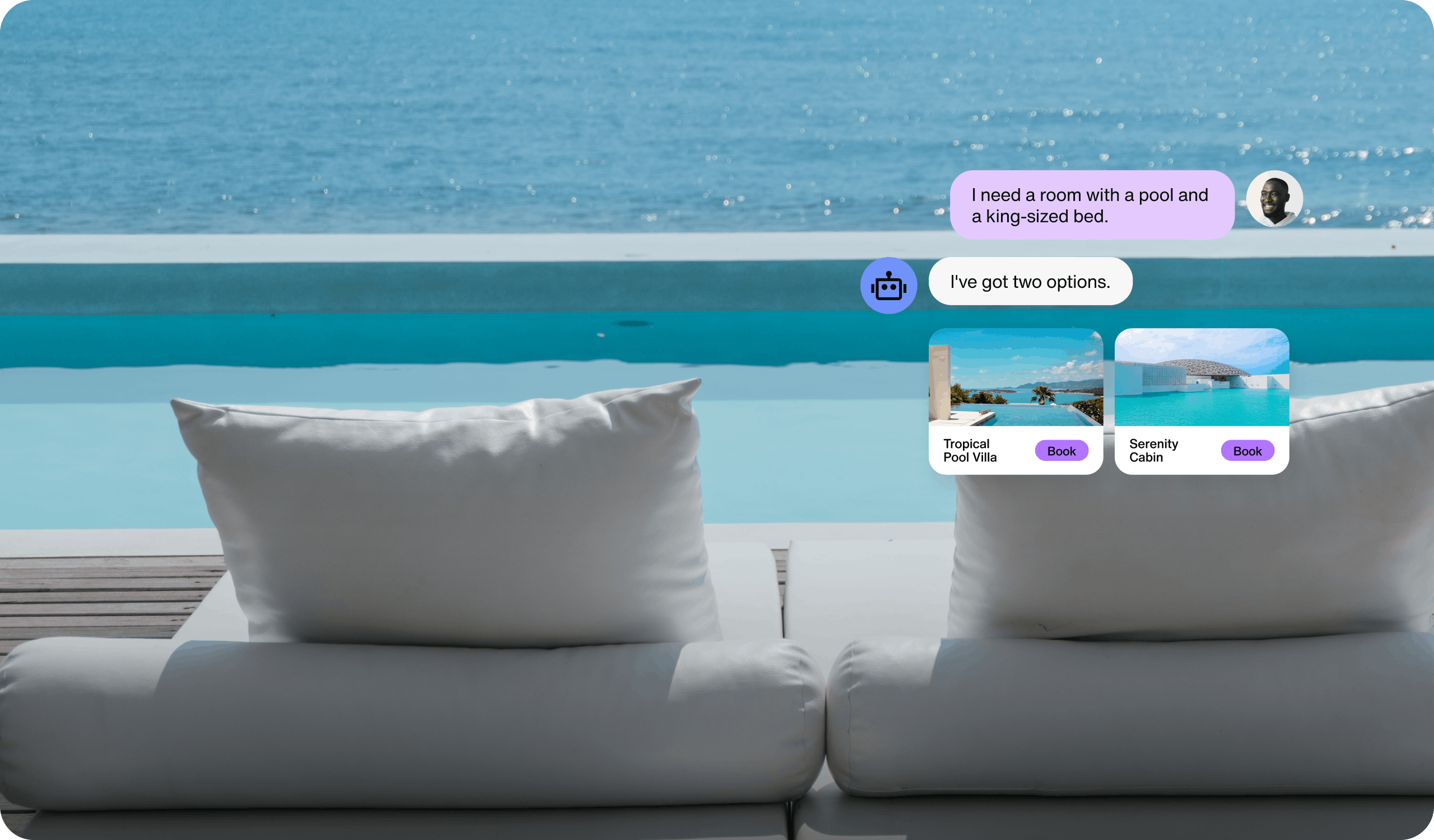
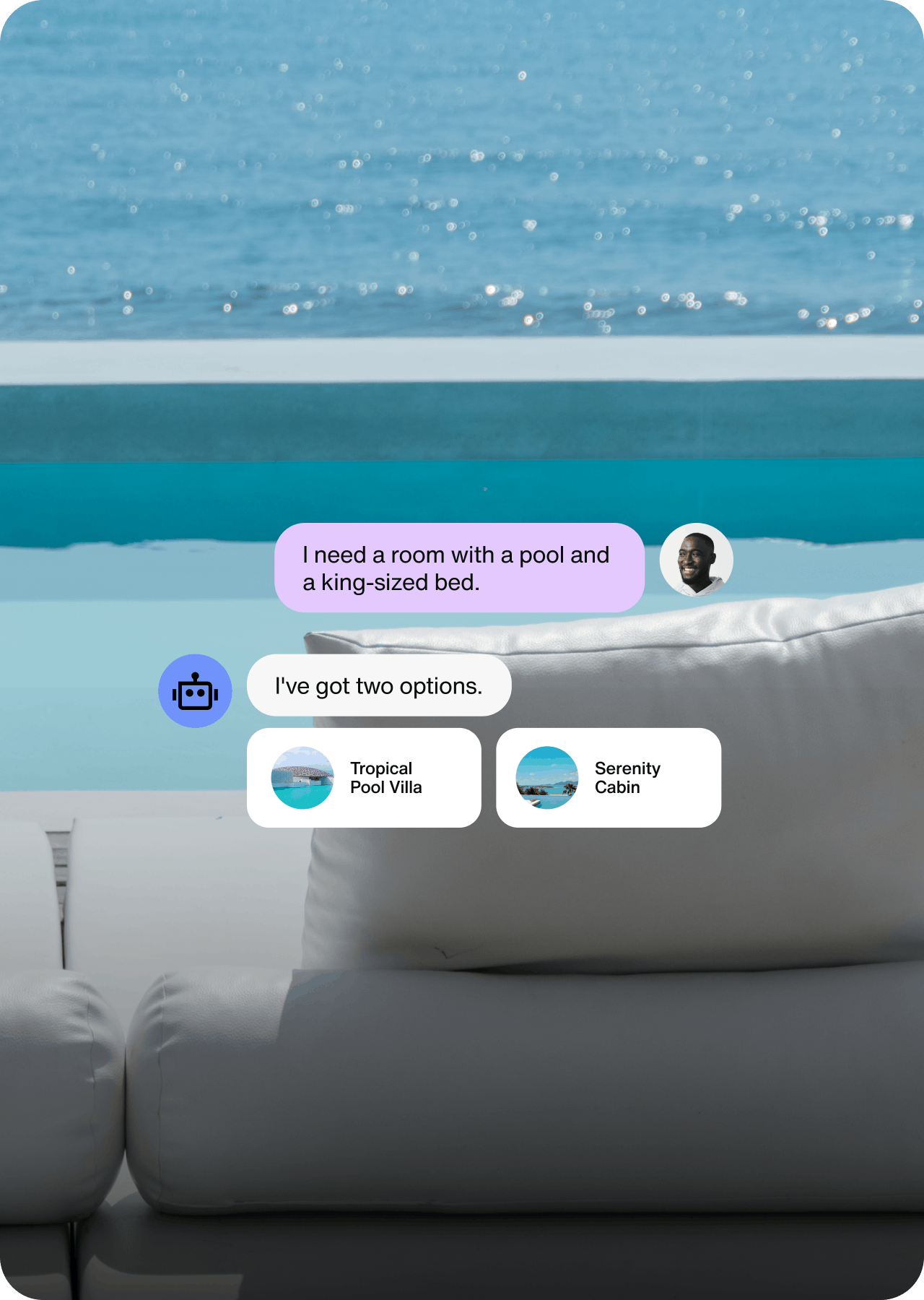
Sendbird helps retailers connect buyers and sellers within the mobile app to increase conversions and engagement, while boosting retention with better support.
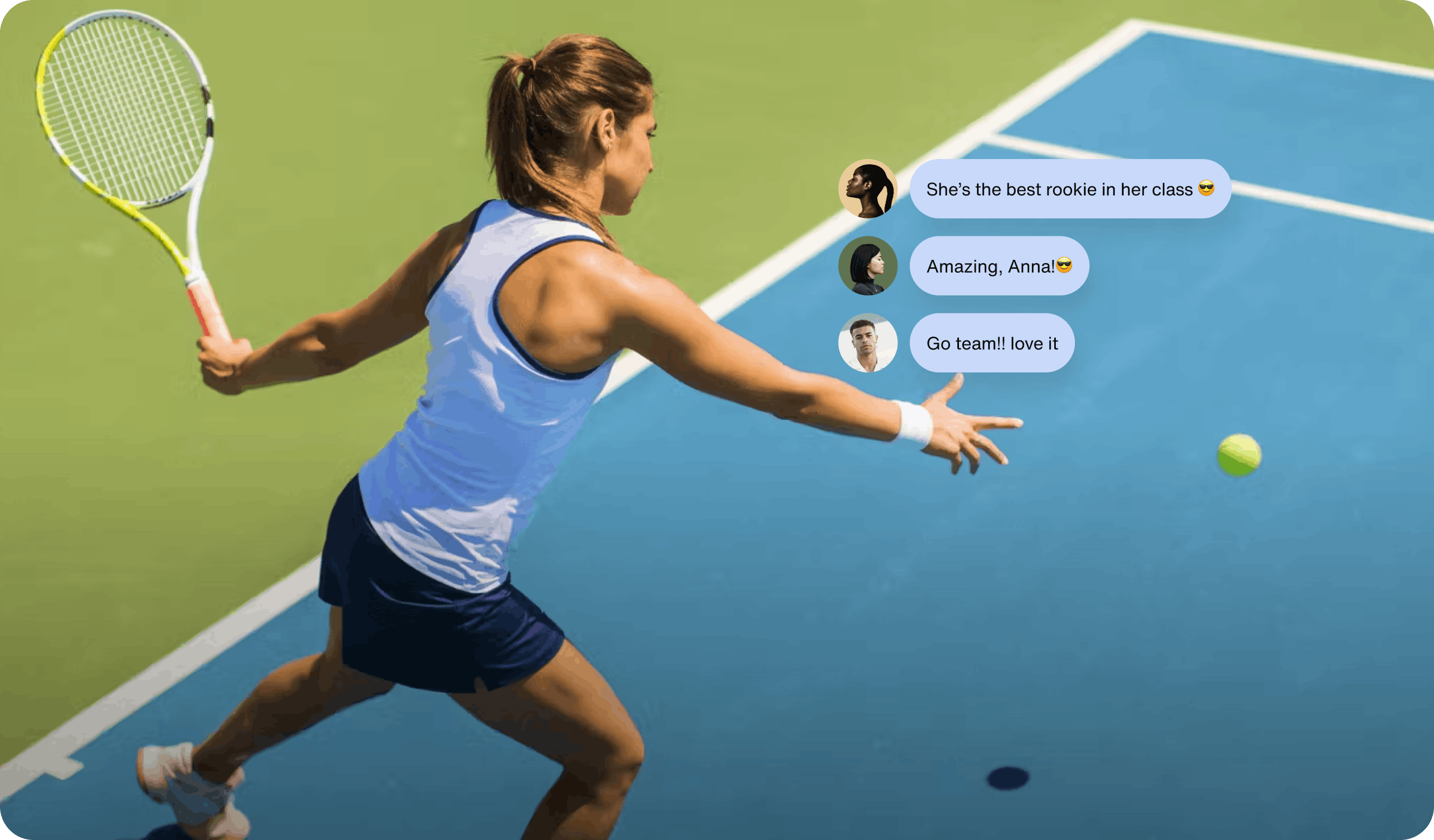
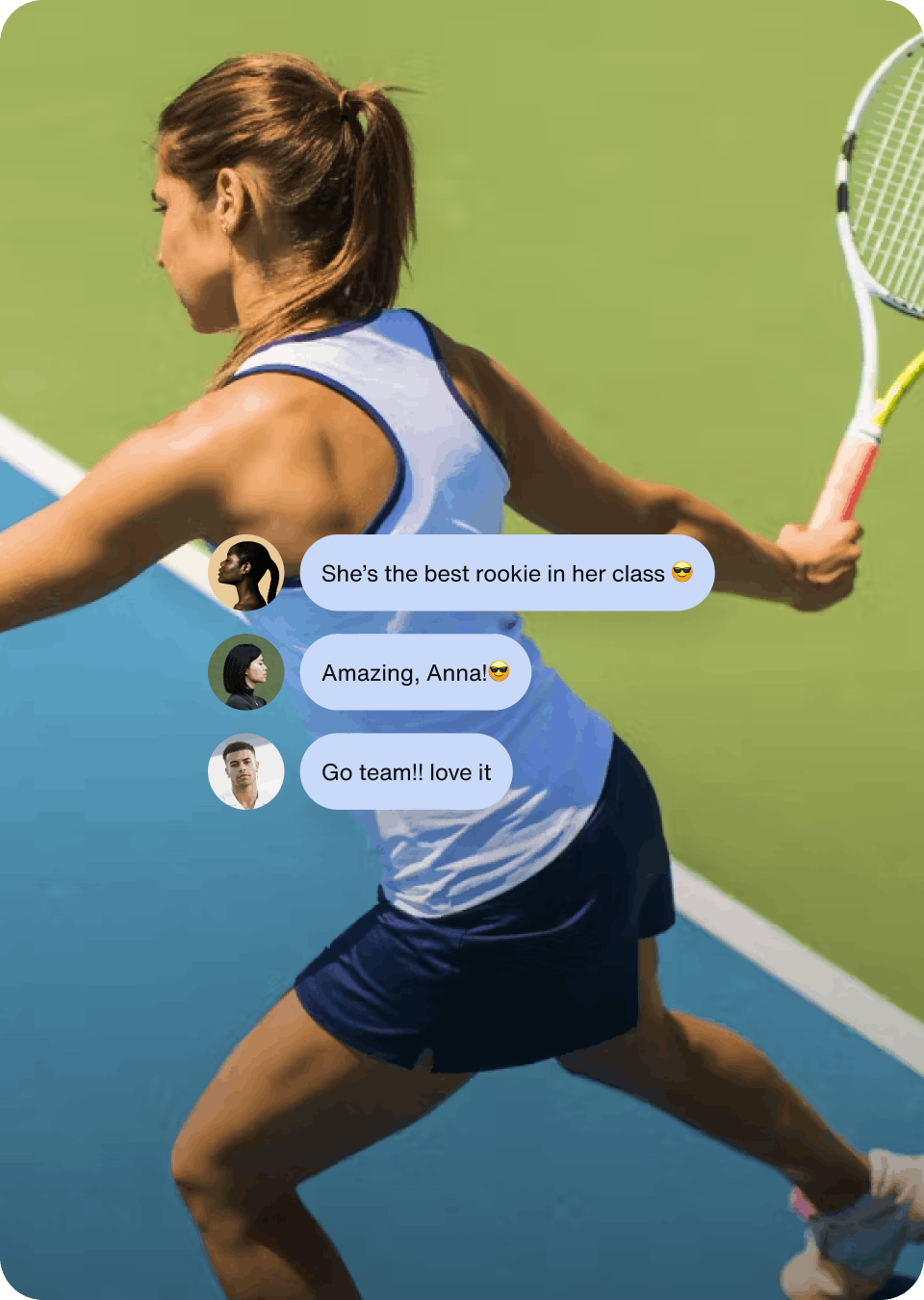
Increase engagement and reduce churn within your community. Keep the conversation safe with essential moderation tools.
Developers come first
Sendbird’s chat API, voice API, video API, native chat SDKs, feature-rich platform, and ready-made UI components make developers more productive. We take care of a ton of operational complexity under the hood, so you can power a rich chat service plus life-like voice and video experiences—without worrying about features, edge cases, reliability, or scale.
Check out our developer resources:
- IOS
- Android
- JavaScript
- Flutter
- .NET
- Unity
- Platform
let initParams = InitParams(applicationId: appId)
SendbirdChat.initialize(params: initParams)
SendbirdChat.addChannelDelegate(self, identifier: delegateId)
SendbirdChat.connect(userId: userId) { user, error in
// Get channel
GroupChannel.getChannel(url: channelURL) { channel, error in
// Send message
channel?.sendUserMessage(message) { message, error in
// Message sent
}
}
}
SendbirdChat.init(
InitParams(APP_ID, applicationContext, useCaching = true),
object : InitResultHandler {
override fun onMigrationStarted() {
Log.i("Application", "Called when there's an update in Sendbird server.")
}
override fun onInitFailed(e: SendbirdException) {
Log.i("Application", "Called when initialize failed. SDK will still operate properly as if useLocalCaching is set to false.")
}
override fun onInitSucceed() {
Log.i("Application", "Called when initialization is completed.")
}
}
)
SendbirdChat.addChannelHandler(handlerId, object : GroupChannelHandler() {
override fun onMessageReceived(channel: BaseChannel, message: BaseMessage) {
// message received
}
}
SendbirdChat.connect(userId) { user, e ->
if (user != null) {
if (e != null) {
// Proceed in offline mode with the data stored in the local database.
// Later, connection is made automatically.
// and can be notified through ConnectionHandler.onReconnectSucceeded().
} else {
// Proceed in online mode.
}
GroupChannel.getChannel(channelUrl) { groupChannel, e ->
if (e != null || groupChannel == null) {
// Handle error.
return@getChannel
}
groupChannel.sendUserMessage(
UserMessageCreateParams(message)
) { userMessage, e ->
}
}
} else {
// Handle error.
}
}
const sb = SendbirdChat.init({
appId : 'YOUR-APP-ID',
modules: [
new GroupChannelModule()
]
});
sb.groupChannel.addGroupChannelHandler(handlerId, new GroupChannelHandler({
onMessageReceived: (channel, message) => {
// message received
}
}));
const user = await sb.connect(userId);
const channel = await sb.groupChannel.getChannel(channelUrl);
channel.sendUserMessage({ message })
.onPending((message) => {
// message is pending to be sent
})
.onSucceeded((message) => {
// message sent
})
.onFailed((err, message) => {
// message not sent
});
void main() async {
SendbirdChat.init(appId: 'APP-ID');
SendbirdChat.addChannelHandler('HANDLER-ID', MyGroupChannelHandler());
runZonedGuarded(() async {
final user = await SendbirdChat.connect('USER-ID');
final groupChannel = await GroupChannel.getChannel('CHANNEL-URL');
groupChannel.sendUserMessage(
UserMessageCreateParams(message: 'MESSAGE'),
handler: (message, e) {
// message sent
},
);
}, (e, s) {
// handle error
});
}
class MyGroupChannelHandler extends GroupChannelHandler {
@override
void onMessageReceived(BaseChannel channel, BaseMessage message) {
// message received
}
}
SendbirdClient.Init(appId);
const SendbirdClient.ChannelHandler channelHandler = new SendbirdClient.ChannelHandler();
channelHandler.OnMessageReceived = (BaseChannel baseChannel, BaseMessage baseMessage) => {
// message received
};
SendbirdClient.AddChannelHandler(handlerId, channelHandler);
SendbirdClient.Connect(userId, (User user, SendbirdException connectException) => {
// get channel
GroupChannel.GetChannel(channelUrl,
(GroupChannel groupChannel, SendbirdException getChannelException) => {
// send message
groupChannel.SendUserMessage(message,
(UserMessage userMessage, SendbirdException sendMessageException) => {
// message sent
});
});
});
SendbirdClient.Init(appId);
const SendbirdClient.ChannelHandler channelHandler = new SendbirdClient.ChannelHandler();
channelHandler.OnMessageReceived = (BaseChannel baseChannel, BaseMessage baseMessage) => {
// message received
};
SendbirdClient.AddChannelHandler(handlerId, channelHandler);
SendbirdClient.Connect(userId, (User user, SendbirdException connectException) => {
// get channel
GroupChannel.GetChannel(channelUrl,
(GroupChannel groupChannel, SendbirdException getChannelException) => {
// send message
groupChannel.SendUserMessage(message,
(UserMessage userMessage, SendbirdException sendMessageException) => {
// message sent
});
});
});
import requests
# Send a message
response = requests.request(
method='POST',
url=f'https://api-{YOUR_APP_ID}.sendbird.com/v3/group_channels/{channel_url}/messages',
headers={
'Api-Token': API_TOKEN,
},
json={
'message_type': 'MESG',
'user_id': 'user-1',
'message': 'Hello Sendbird!',
},
)
Digital connections drive real results
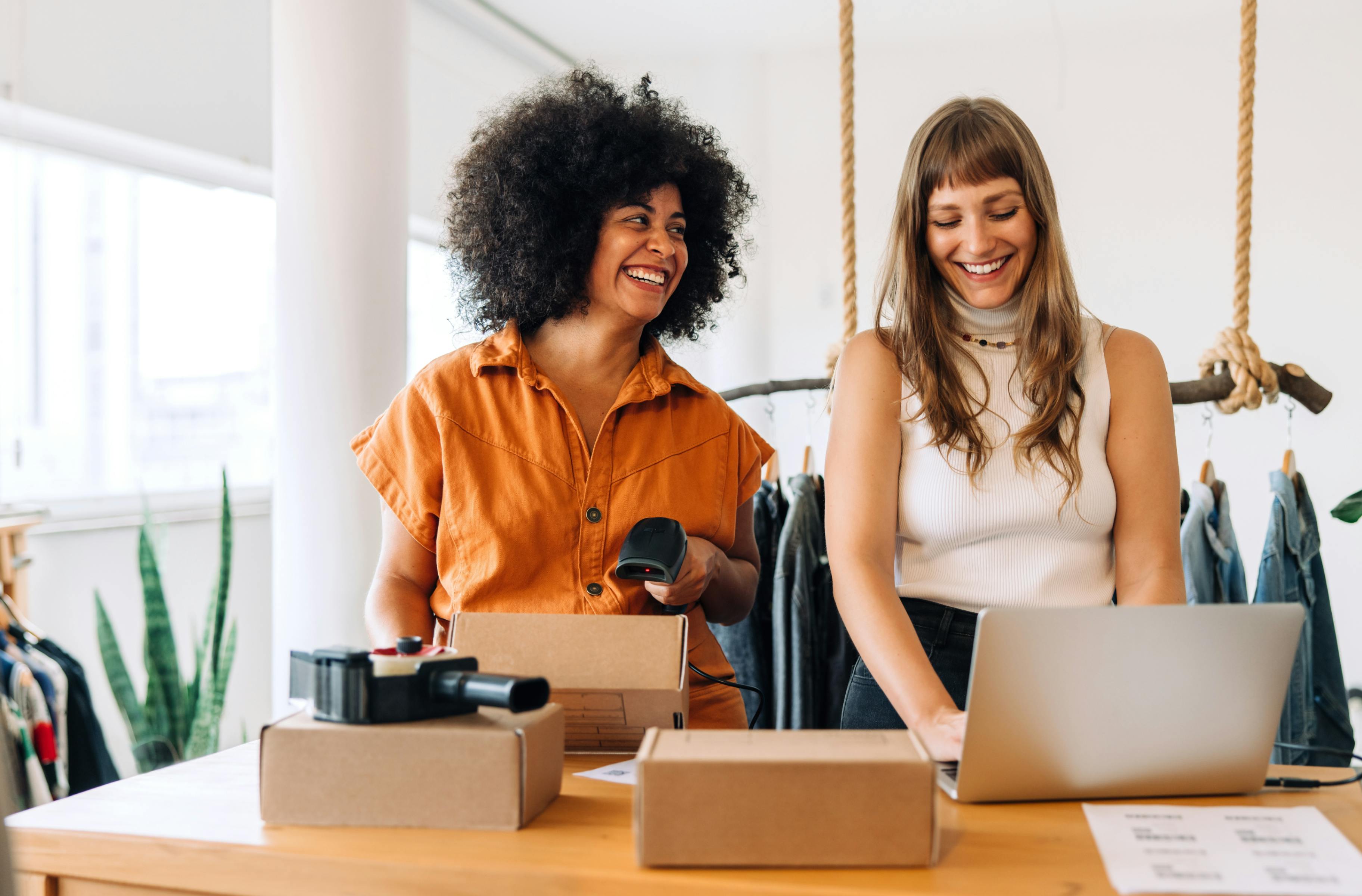
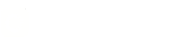
“Sendbird powers our core transaction flows via a fast and reliable messaging layer between our buyers and sellers.”
Carousell, Head of Engineering
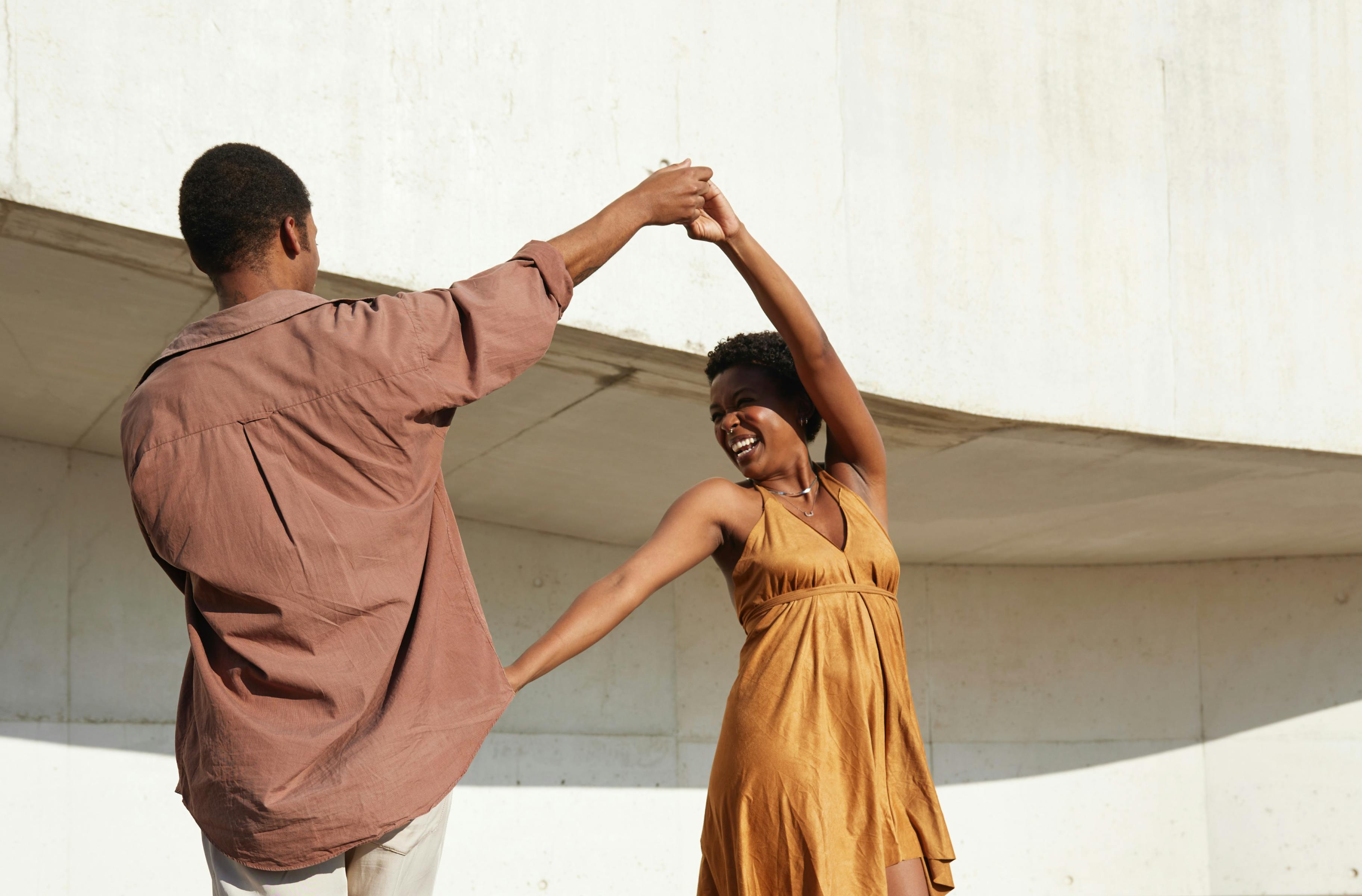
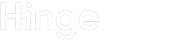
“Sendbird’s client base gave us confidence that they would be able to handle our traffic and projected growth.”
Hinge, CTO
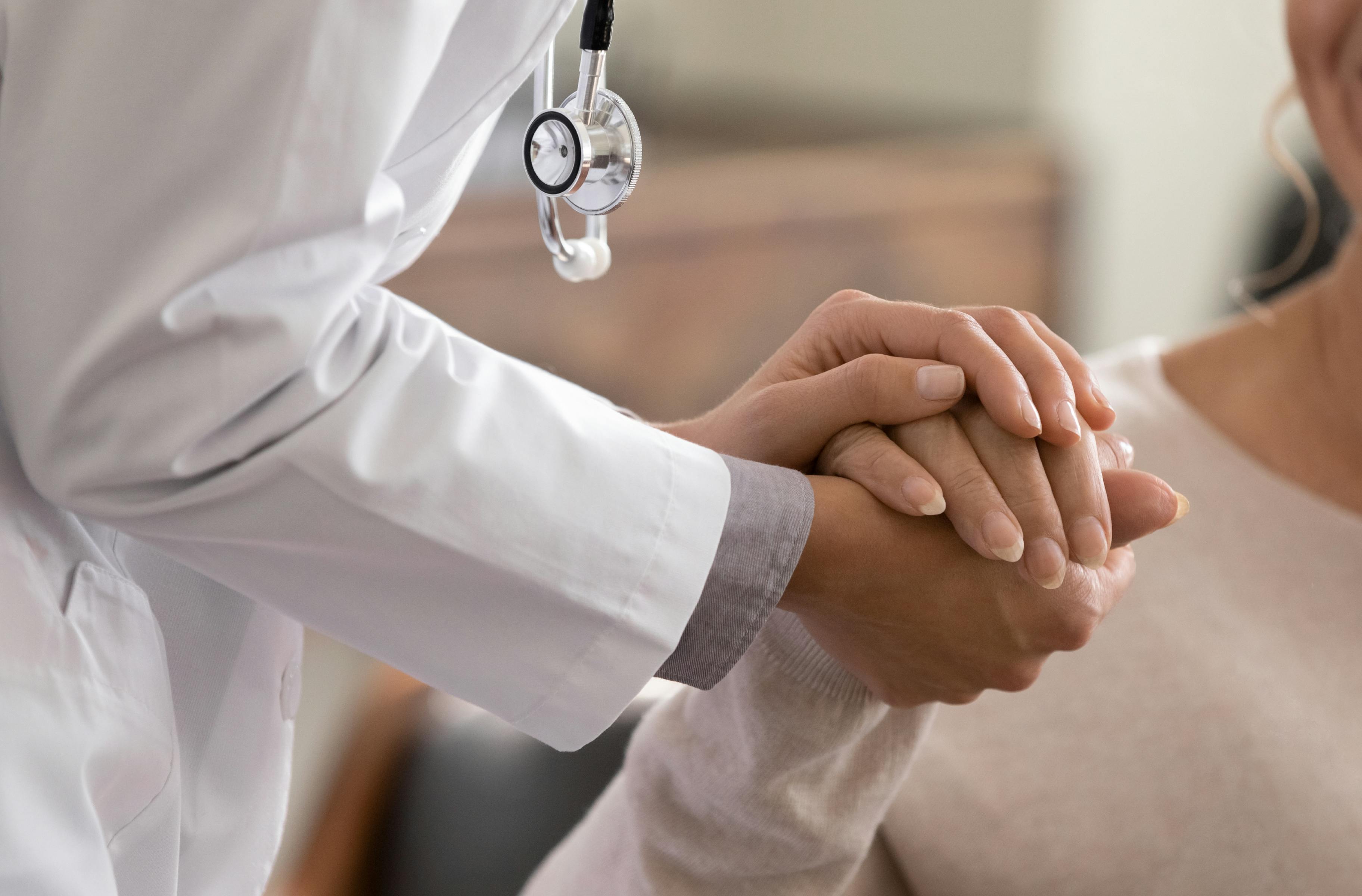
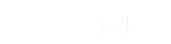
“With Sendbird's developer-friendly chat API and SDKs we were quickly able to build in-app chat.”
Accolade, Director of Product Management
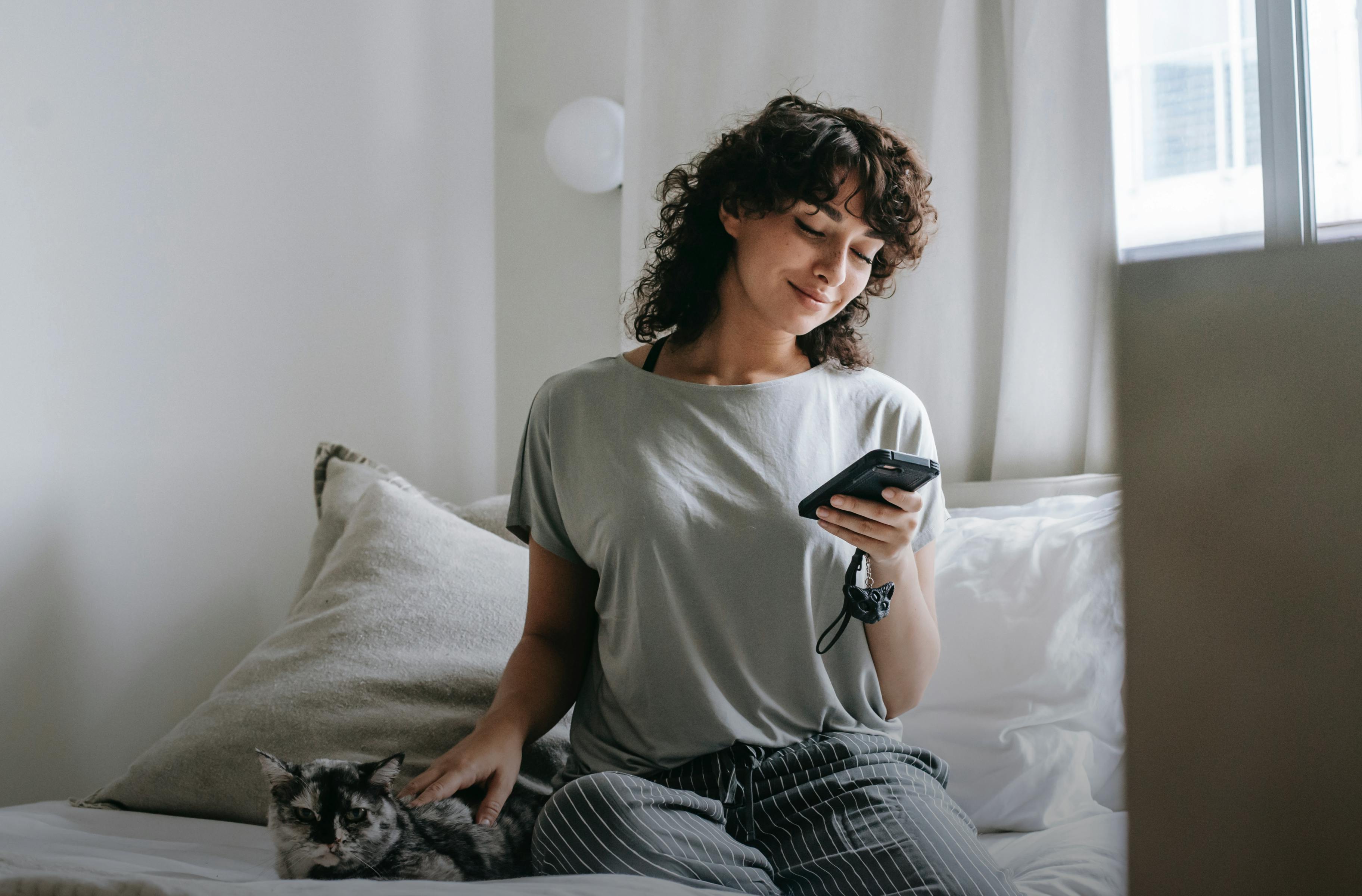
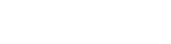
“With Sendbird we provide a 100% digital support experience for our customers with native in-app chat based interactions.”
Virgin Mobile UAE, Senior Manager
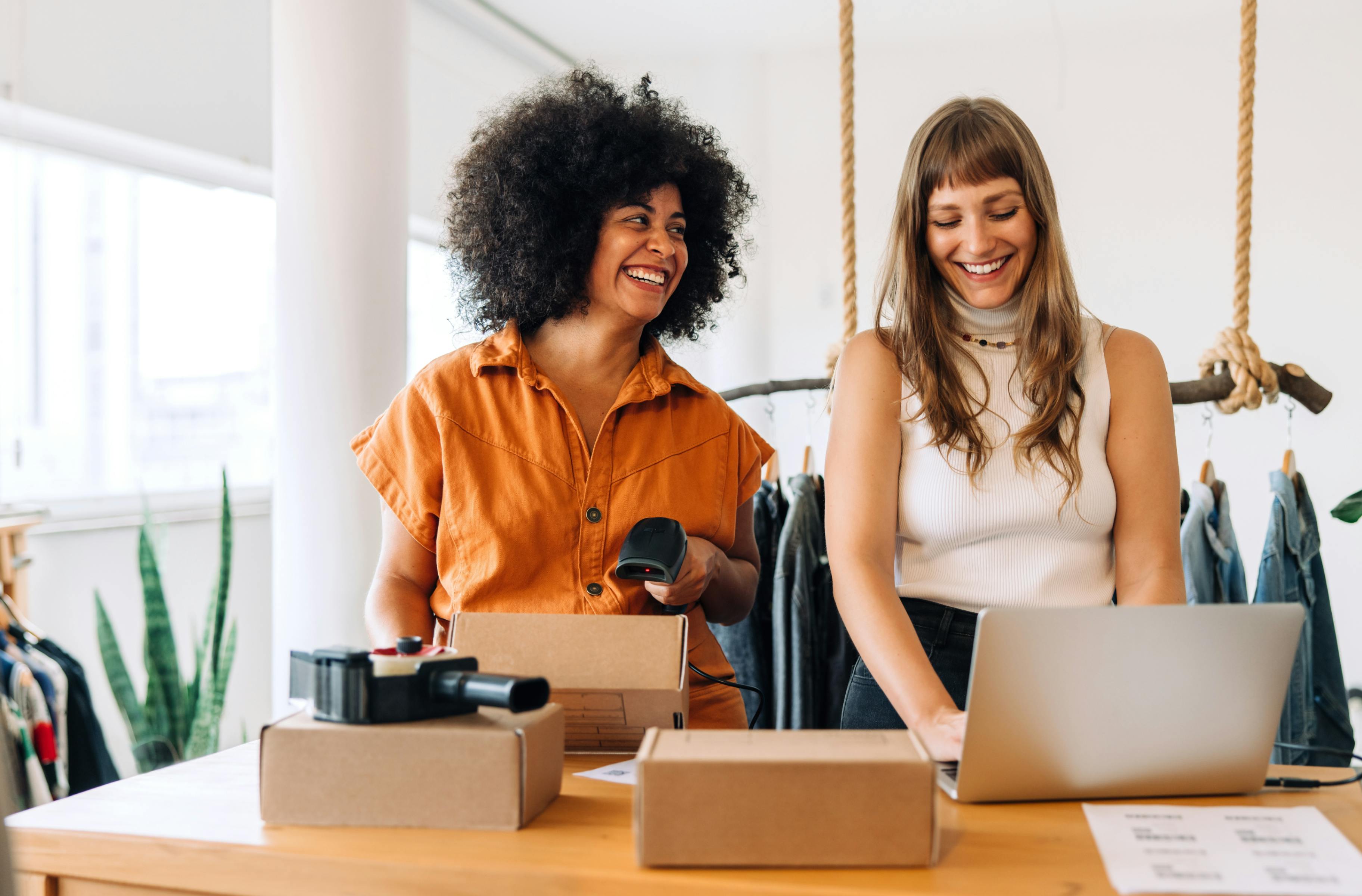
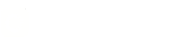
“Sendbird powers our core transaction flows via a fast and reliable messaging layer between our buyers and sellers.”
Carousell, Head of Engineering
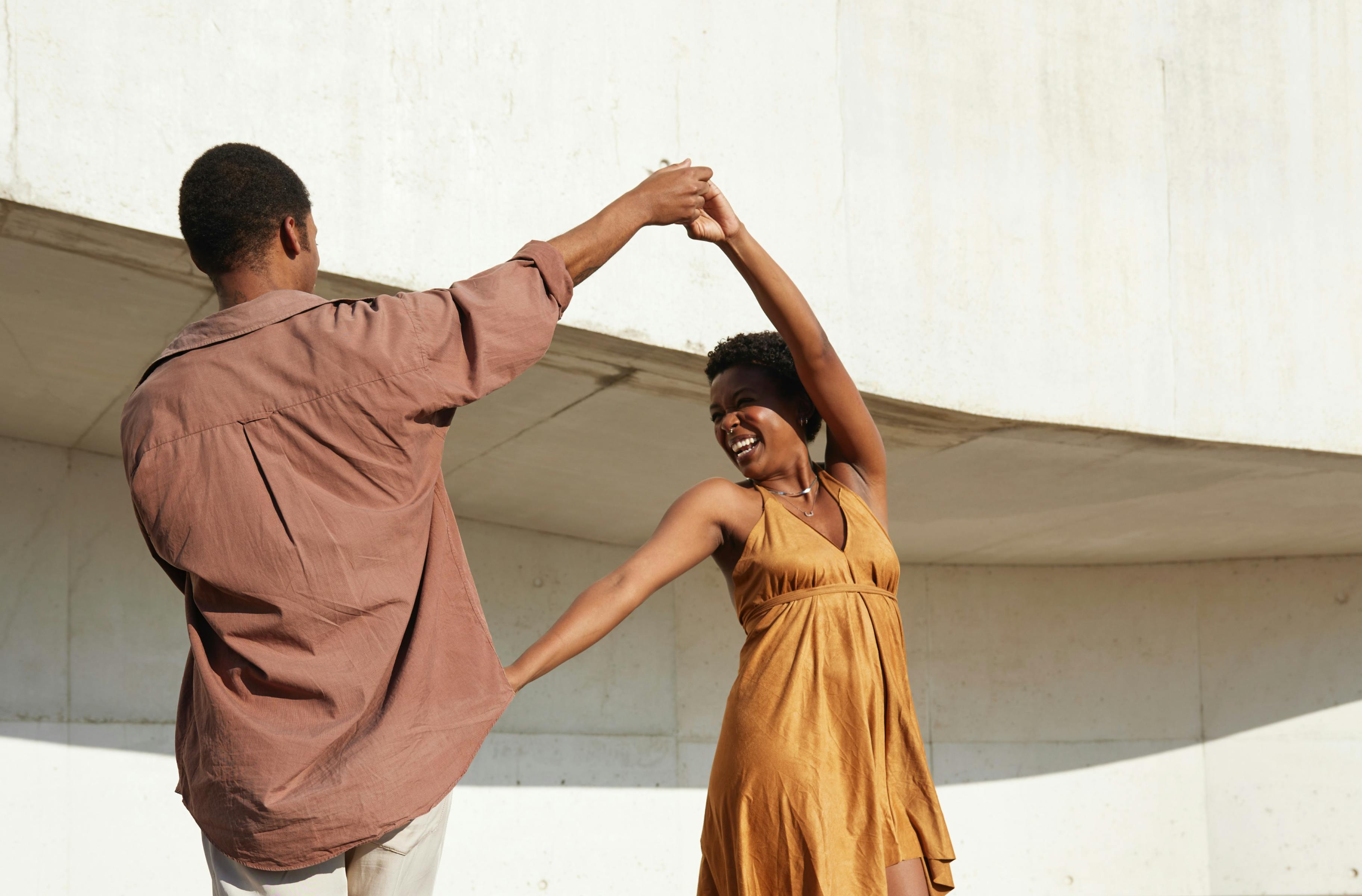
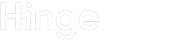
“Sendbird’s client base gave us confidence that they would be able to handle our traffic and projected growth.”
Hinge, CTO
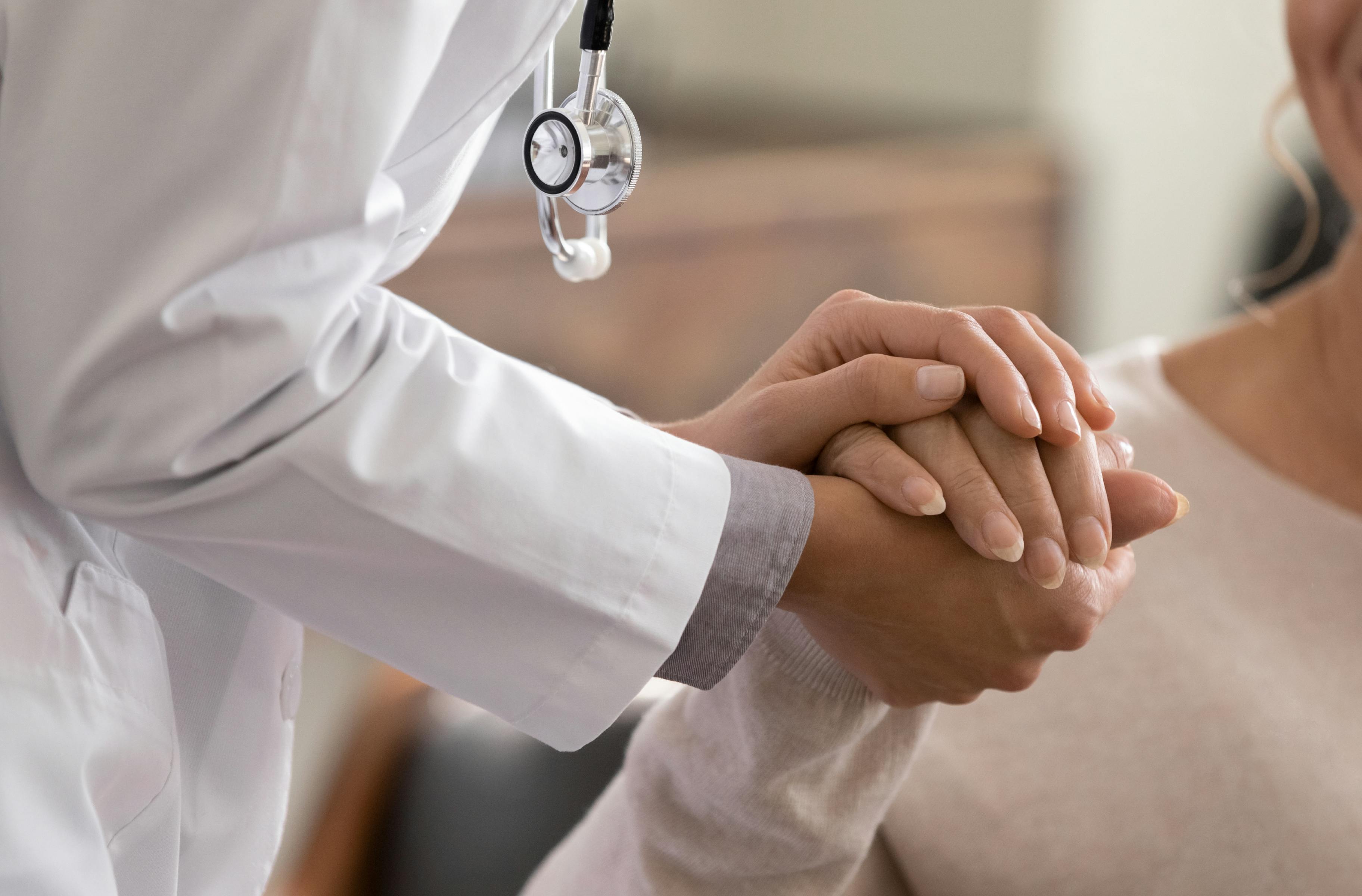
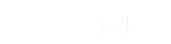
“With Sendbird's developer-friendly chat API and SDKs we were quickly able to build in-app chat.”
Accolade, Director of Product Management
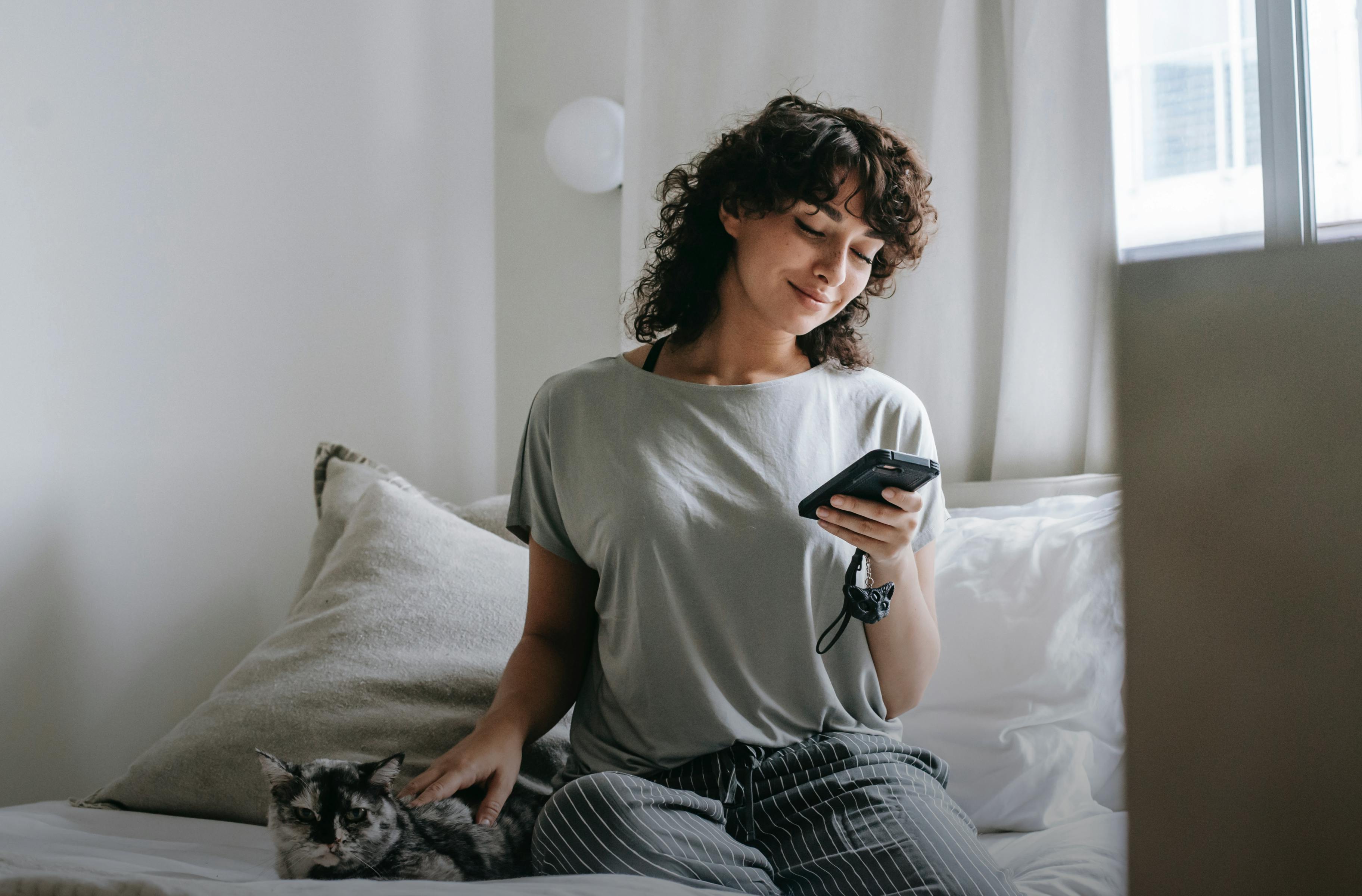
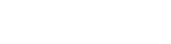
“With Sendbird we provide a 100% digital support experience for our customers with native in-app chat based interactions.”
Virgin Mobile UAE, Senior Manager
Enterprise-ready compliance
The fastest growing startups to the largest enterprises trust Sendbird with their data. Our chat API, voice API, video API, and conversations platform adhere to leading security and compliance standards to ensure that all your communication and data are encrypted both in rest and motion.