How to build in-app chat using Kotlin – Part 1
Introduction
This tutorial is an easy-to-follow Kotlin implementation guide to build a chat application using the Sendbird Chat SDK. The complete tutorial includes two parts. After completing it, you will have created your very own chat application with Kotlin.
In this first part, we’ll go over the initialization and login, and the display, selection, and creation of channels. Sendbird has two channel types: Open Channels and Group Channels. This guide focuses on the Group Channel implementation to create 1:1 private chats and private group chats.
We’ll create three activities:
- LoginActivity – A basic Login screen for users to log in and set their nickname
- ChannelListActivity – A channel list to display the Group Channels that a user can join
- ChannelCreationActivity – A screen that allows a user to invite other users and create a channel
Then we’ll cover the following steps:
- Import dependencies and add permissions
- Login Activity, including:
- Creating a basic Login UI
- Create LoginActivity
- Displaying a channel list, including:
- Create a channel list UI
- Create ChannelListActivity
- Create ChannelListAdapter for RecyclerView
- Creating Channels, including:
- Implement a Channel Create UI
- Implement ChannelCreateActivity
- Implement ChannelCreateAdapter for RecyclerView
Please note that this tutorial assumes a basic familiarity with Kotlin.
Let’s get started!
Step 1. Import dependencies and add permissions
First, add the most recent Sendbird SDK to the app level build.gradle file.
Step 2. Login Activity
To begin chatting, a user must be able to log in or create a user.
This section covers two parts, including how to:
- Initialize the Sendbird application with your respective AppID
- Connect to Sendbird with a UserID, and nickname
But first, we need to create a basic login UI.
- The basic sign-in UI includes an Edit Text field for the UserID, a field for the desired nickname, and a button to connect. See the image below.
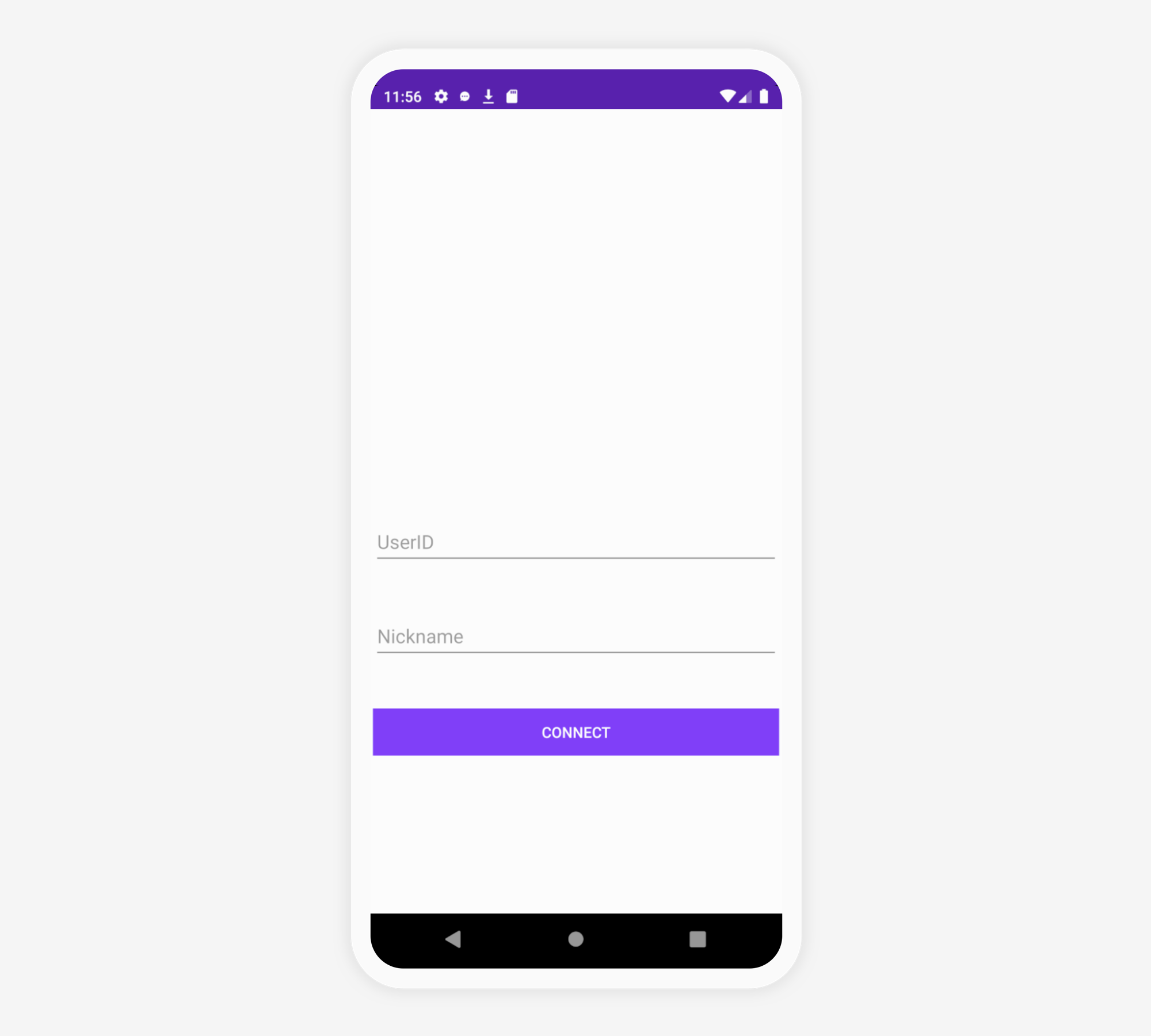
You can view the full code here:
activity_login.xml
Create LoginActivity
Create a new Activity called LoginActivity.kt. Be sure to register this activity in the manifest. This activity is responsible for initializing and handling the connection to your Sendbird application.
Ideally, you would choose to put the initialization in a different activity. For the sake of simplicity, we’ll initialize in the LoginActivity.
Add an onCreate method. This method handles both the UI elements and the initialization process.
onCreate method in LoginActivity.kt
The connectToSendBird() method connects a passed UserID to Sendbird and then updates the Nickname of the connected user. Upon completion, it launches our ChannelListActivity.
connectToSendBird() method in LoginActivity.kt
See the following gist for the complete contents of LoginActivity.kt.
Step 3. Displaying a channel list
Once you’ve created the login, create an Activity to display, choose, and create channels. The following shows you how to query a list of channels associated with the user and render and display them in a RecyclerView.
Create a channel list UI
Create a basic item for the channel list RecyclerView. For simplicity, we’ll create a CardView with TextViews for the channel name, member count, and most recent message.
item_channel_chooser.xml
Then, create an actual view that contains the RecyclerView for the Channel List. This view also contains a FloatingActionButton, which enables users to create channels.
activity_channel.xml
Create ChannelListActivity
Next, create a new Activity called ChannelListActivity. Make sure to register this Activity in the manifest. This Activity is responsible for adding channels to the RecyclerView, handling the UI interaction when a user creates a new channel and launching the next Activity with the chosen channel.
The onCreate method sets the layout, sets up the RecyclerView, and contains onClickListener for the FloatingActionButton that launches the ChannelCreateActivity.
onCreate() method in ChannelListActivity.kt
The onCreate method calls a method addChannels(). This method creates a query that retrieves a specified range of channels. Retrieve more channels by checking the .hasNext() call on the query object. After the result returns, it’s possible to add the channels to the Adapter.
addChannels() method in ChannelListActivity.kt
The onItemClicked() method overrides a method call from the interface that you will implement in the adapter. It allows you to set a click listener on an individual view. Inside this method, you will need to create an intent to start the ChannelActivity with the channel.URL. The second part of this guide will cover the creation of ChannelActivity and handling the intent to start ChannelActivity.
onItemClicked in ChannelListActivity.kt
To see the full implementation of this class, see the gist below:
Create ChannelListAdapter for RecyclerView
Create an adapter to populate the RecyclerView with the returned channel list. This adapter populates the views and adds an onClickListener to each view, launching the chat Activity for a respective channel.
The guide breaks this class into two parts:
- The standard implementation of an adapter, ChannelListAdapter, for the RecyclerView
- An inner class, ChannelHolder, that implements the ViewHolder pattern
See the following gist for the first part, i.e. the adapter that populates the RecyclerView with the returned channel list
ChannelListAdapter:
The second part of this class involves an inner class that implements the ViewHolder pattern. This binds data to the corresponding views. In the bindViews method, you can see that we pass a groupChannel object and an OnChannelClickedListener. These two objects set up the views. Make sure to set the onClickListener on the itemView to the listener passed in the bindViews method.
ChannelHolder in ChannelListAdapter:
See the full implementation of ChannelListAdapter.kt in the gist below:
Step 4. Creating channels
This section covers how to query a list of users, display those users, and create a channel with that list of users.
Implement a Channel Create UI
As before, create a layout with a RecyclerView and a simple button.
Activity_create.xml
Create an item that will hold the content of a user. To do so, create a simple layout that contains a CheckBox, with a TextView.
Item_create.xml
Create another Activity and call it ChannelCreateActivity. Make sure to register this activity in the manifest. This Activity loads the list of users, sets up the UI elements and the RecyclerView adapter, and handles the creation of channels.
The onCreate method sets up the necessary components. In the full code of ChannelCreateActivity, initialize a global variable called selectedUsers, which will be the list of users you pass when creating a channel. Set up your RecyclerView and Adapter. After that call, see the method loadUsers(), implemented below. Finally, set an onClickListener for when a user opts to create a channel.
onCreate() in ChannelCreateActivity.kt
Now, implement the loadUsers() method. The example uses the default generic query to get the application’s users. As in channel querying, it’s possible to customize what to return by setting certain variables on the userListQuery. When the query returns, add the returned users to your adapter.
loadUsers() in ChannelCreateActivity.kt
Like before, add an interface to our adapter class to handle actions on individual items in the RecyclerView. This interface will have one method, onItemChecked(), implemented below. It indicates whether a user has been checked or not, and updates the selectedUsers list accordingly.
onItemChecked() in ChannelCreateActivity.kt
Finally, implement the createChannel() method. As its name implies, this method creates the channel with the passed users. When creating a channel in Sendbird, there’s a lot of freedom to customize the channel. By creating a params val, you can add users, add operators (Admins), set the channel name, and so on. Once you are done specifying your optional params, create a Group Channel with the passed params. Once it returns, create an intent to launch ChannelActivity with the Channel URL as an ‘Extra’.
createChannel() in ChannelCreateActivity.kt
Here is the full implementation of ChannelCreateActivity.
ChannelCreateActivity.kt
Implement ChannelCreateAdapter for RecyclerView
The last thing to implement is the custom adapter for our RecyclerView.
As before, the guide divides this into two sections:
- The standard implementation of an adapter, ChannelCreateAdapter, for RecyclerView
- An inner class, UserHolder, that implements the ViewHolder pattern
The ChannelCreateAdapter part covers the basic approach to an Adapter implementation. This requires an interface for when an item gets checked and a SparseBooleanArray() to handle when Users are checked/unchecked. Other than these two requirements, follow the standard approach of implementing the required methods.
ChannelCreateAdapter
Create an inner class that extends RecyclerView.ViewHolder. This class binds the views from item_create.xml. It will also be responsible for handling checking and unchecking of the CheckBoxes, and updating the list of users respectively.
UserHolder
See the gist below for a full implementation of ChannelCreateAdapter.kt
Conclusion
You are halfway to creating your own chat application with Kotlin!
In this first part of the Kotlin implementation guide, we covered how to:
- Import dependencies and add permissions
- Create LoginActivity and a basic Login UI
- Create ChannelListActivity, ChannelListAdapter for RecyclerView, and a Channel List UI
- Implement ChannelCreateActivity, ChannelCreateAdapter for RecyclerView, and a Channel Create UI
You can now check out part-2 of the Kotlin guide! In part 2, you will complete your own chat application by adding a chat UI and enabling users to send and receive messages. For questions, remember to check our community site and consult our docs.
You are well on your way to creating an engaging Android mobile app. Keep going, and happy mobile chat building!